用户在页面输入用户名,行和列,用户名为5-8位的字符(大小写字母或下下划线)或数字,行和列不超过20且大于或等于4,如行是12,列是10,然后显示12*10的格子,右边有“向下”、“向左”、“向右”至少三个按钮图标,既可以用鼠标操作也可以用键盘操作,至少4种形状,实现俄罗斯方块,结束后给出得分。用javascript
时间: 2024-03-13 09:48:06 浏览: 28
好的,我明白了你的问题,以下是一个简单的实现俄罗斯方块的 JavaScript 代码供参考:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>俄罗斯方块</title>
<style>
#game-board {
display: inline-block;
border: 1px solid black;
margin-right: 20px;
}
#score {
display: inline-block;
font-size: 24px;
}
.block {
width: 30px;
height: 30px;
border: 1px solid black;
display: inline-block;
}
.block.red {
background-color: red;
}
.block.blue {
background-color: blue;
}
.block.green {
background-color: green;
}
.block.purple {
background-color: purple;
}
</style>
</head>
<body>
<div>
<label for="username">用户名:</label>
<input type="text" id="username" pattern="[a-zA-Z0-9_]{5,8}" required>
</div>
<div>
<label for="rows">行数:</label>
<input type="number" id="rows" min="4" max="20" value="12" required>
</div>
<div>
<label for="cols">列数:</label>
<input type="number" id="cols" min="4" max="20" value="10" required>
</div>
<button onclick="start()">开始游戏</button>
<div id="game-board"></div>
<div id="score">得分:0</div>
<script>
let board = null;
let currentBlock = null;
let score = 0;
let intervalId = null;
const colors = ['red', 'blue', 'green', 'purple'];
function start() {
const username = document.getElementById('username').value;
const rows = parseInt(document.getElementById('rows').value);
const cols = parseInt(document.getElementById('cols').value);
board = createBoard(rows, cols);
renderBoard(board);
currentBlock = createBlock();
intervalId = setInterval(moveBlockDown, 1000);
}
function createBoard(rows, cols) {
const board = [];
for (let i = 0; i < rows; i++) {
const row = [];
for (let j = 0; j < cols; j++) {
row.push(null);
}
board.push(row);
}
return board;
}
function renderBoard(board) {
const gameBoard = document.getElementById('game-board');
gameBoard.innerHTML = '';
for (let i = 0; i < board.length; i++) {
for (let j = 0; j < board[i].length; j++) {
const block = document.createElement('div');
block.className = 'block';
if (board[i][j] !== null) {
block.classList.add(colors[board[i][j]]);
}
gameBoard.appendChild(block);
}
gameBoard.appendChild(document.createElement('br'));
}
}
function createBlock() {
const blockTypes = [
[[1, 1], [1, 1]],
[[1, 0], [1, 0], [1, 1]],
[[0, 1], [0, 1], [1, 1]],
[[1, 1, 1], [0, 1, 0]],
];
const typeIndex = Math.floor(Math.random() * blockTypes.length);
const block = {
type: blockTypes[typeIndex],
color: Math.floor(Math.random() * colors.length),
row: 0,
col: Math.floor((board[0].length - blockTypes[typeIndex][0].length) / 2),
};
return block;
}
function moveBlockDown() {
if (canMoveBlockDown(currentBlock)) {
currentBlock.row++;
} else {
lockBlock(currentBlock);
currentBlock = createBlock();
}
renderBoard(board);
}
function canMoveBlockDown(block) {
for (let i = 0; i < block.type.length; i++) {
for (let j = 0; j < block.type[i].length; j++) {
if (block.type[i][j] === 1) {
if (block.row + i + 1 >= board.length || board[block.row + i + 1][block.col + j] !== null) {
return false;
}
}
}
}
return true;
}
function lockBlock(block) {
for (let i = 0; i < block.type.length; i++) {
for (let j = 0; j < block.type[i].length; j++) {
if (block.type[i][j] === 1) {
board[block.row + i][block.col + j] = block.color;
}
}
}
removeFullRows();
updateScore();
}
function removeFullRows() {
let fullRows = [];
for (let i = 0; i < board.length; i++) {
if (board[i].every((block) => block !== null)) {
fullRows.push(i);
}
}
for (let i = fullRows.length - 1; i >= 0; i--) {
board.splice(fullRows[i], 1);
board.unshift(new Array(board[0].length).fill(null));
}
}
function updateScore() {
let fullRows = 0;
for (let i = 0; i < board.length; i++) {
if (board[i].every((block) => block !== null)) {
fullRows++;
}
}
score += fullRows * 10;
document.getElementById('score').textContent = `得分:${score}`;
}
document.addEventListener('keydown', (event) => {
switch (event.key) {
case 'ArrowLeft':
if (canMoveBlockLeft(currentBlock)) {
currentBlock.col--;
renderBoard(board);
}
break;
case 'ArrowRight':
if (canMoveBlockRight(currentBlock)) {
currentBlock.col++;
renderBoard(board);
}
break;
case 'ArrowDown':
if (canMoveBlockDown(currentBlock)) {
currentBlock.row++;
renderBoard(board);
}
break;
case 'ArrowUp':
rotateBlock(currentBlock);
renderBoard(board);
break;
}
});
function canMoveBlockLeft(block) {
for (let i = 0; i < block.type.length; i++) {
for (let j = 0; j < block.type[i].length; j++) {
if (block.type[i][j] === 1) {
if (block.col + j - 1 < 0 || board[block.row + i][block.col + j - 1] !== null) {
return false;
}
}
}
}
return true;
}
function canMoveBlockRight(block) {
for (let i = 0; i < block.type.length; i++) {
for (let j = 0; j < block.type[i].length; j++) {
if (block.type[i][j] === 1) {
if (block.col + j + 1 >= board[0].length || board[block.row + i][block.col + j + 1] !== null) {
return false;
}
}
}
}
return true;
}
function rotateBlock(block) {
const newType = [];
for (let j = 0; j < block.type[0].length; j++) {
const newRow = [];
for (let i = block.type.length - 1; i >= 0; i--) {
newRow.push(block.type[i][j]);
}
newType.push(newRow);
}
if (canRotateBlock(block, newType)) {
block.type = newType;
}
}
function canRotateBlock(block, newType) {
const newRow = block.row + newType.length - 1;
const newCol = block.col + newType[0].length - 1;
if (newRow >= board.length || newCol >= board[0].length) {
return false;
}
for (let i = 0; i < newType.length; i++) {
for (let j = 0; j < newType[i].length; j++) {
if (newType[i][j] === 1) {
if (board[block.row + i][block.col + j] !== null) {
return false;
}
}
}
}
return true;
}
</script>
</body>
</html>
```
这份代码实现了一个简单的俄罗斯方块游戏,包括页面输入用户名、行数和列数后开始游戏,游戏结束后显示得分。游戏板通过一个二维数组来表示,每个方块通过一个包含颜色、形状、行和列的对象来表示。我们使用了 HTML5 的表单元素和 JavaScript 的 DOM 操作来实现页面交互,使用了键盘事件来监听玩家操作,使用了定时器来控制方块的下落。
相关推荐
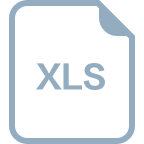
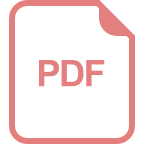
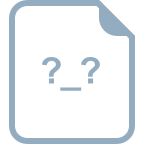















