public Model() { points = new ArrayList<Point>(); listeners = new ArrayList<ModelListener>(); // Read points from file if it exists File file = new File("points.txt"); if (file.exists()) { try { Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String[] coordinates = scanner.nextLine().split(","); int x = Integer.parseInt(coordinates[0]); int y = Integer.parseInt(coordinates[1]); points.add(new Point(x, y)); } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } } },, public void saveData() { try { FileWriter writer = new FileWriter("points.txt"); for (Point p : points) { writer.write(p.x + " " + p.y + "\n"); } writer.close(); } catch (IOException e) { e.printStackTrace(); } }出现以下问题:Exception in thread "main" java.lang.NumberFormatException: For input string: "119.0"
时间: 2024-01-04 12:02:35 浏览: 50
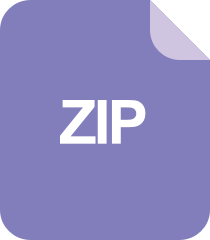
intent传递ArrayList<T>
这个问题可能是因为在读取文件时,将字符串转换为整数时出现了错误。具体来说,您正在尝试将包含小数点的字符串“119.0”转换为整数,这是不可能的,因此会引发NumberFormatException异常。
您需要检查文件中每个坐标是否都是整数,如果不是,则需要更改读取坐标的代码以处理小数点。您还可以使用Double.parseDouble()方法将字符串转换为double类型,然后将其转换为整数。
阅读全文
相关推荐
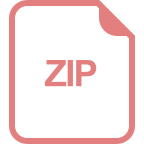
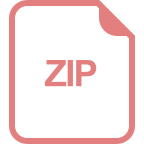







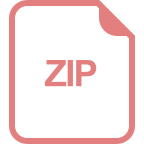
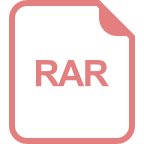
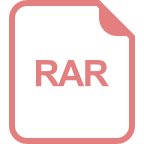
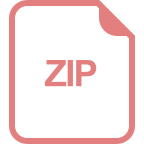
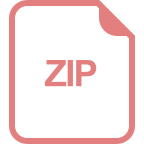
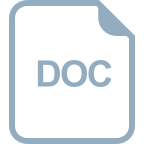
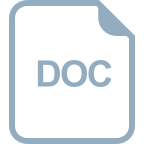
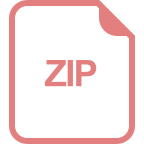