用python绘制倒立摆动画的代码,绘制红色的小球和蓝色的连杆
时间: 2024-05-12 17:19:55 浏览: 15
以下是用Python绘制倒立摆动画的代码,绘制红色的小球和蓝色的连杆:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# 定义常数
g = 9.81 # 重力加速度 m/s^2
L = 1.0 # 摆杆长度 m
m = 1.0 # 摆球质量 kg
b = 0.1 # 摩擦系数
theta0 = np.pi / 4 # 初始角度
omega0 = 0.0 # 初始角速度
# 定义运动方程
def pendulum(y, t, g, L, m, b):
theta, omega = y
dydt = [omega, -b/m*omega - g/L*np.sin(theta)]
return dydt
# 定义初始状态
y0 = [theta0, omega0]
# 定义时间范围和时间步长
t = np.linspace(0, 10, 1000)
# 求解微分方程
sol = odeint(pendulum, y0, t, args=(g, L, m, b))
# 绘制图形
x = L * np.sin(sol[:, 0])
y = -L * np.cos(sol[:, 0])
fig = plt.figure()
ax = fig.add_subplot(111, aspect='equal')
ax.set_xlim(-1.5, 1.5)
ax.set_ylim(-1.5, 1.5)
ax.set_xlabel("x (m)")
ax.set_ylabel("y (m)")
ax.set_title("Inverted Pendulum Simulation")
line, = ax.plot([], [], '-b', lw=2)
point, = ax.plot([], [], 'ro', markersize=10)
def init():
line.set_data([], [])
point.set_data([], [])
return line, point,
def animate(i):
line.set_data([0, x[i]], [0, y[i]])
point.set_data([x[i]], [y[i]])
return line, point,
from matplotlib.animation import FuncAnimation
ani = FuncAnimation(fig, animate, frames=len(t), init_func=init, blit=True)
plt.show()
```
运行后将会得到一个倒立摆的动画,其中红色小球表示摆球,蓝色连杆表示摆杆。可以通过修改常数和初始状态来调整动画的表现。
相关推荐
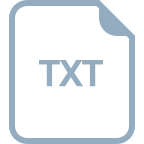
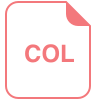
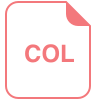
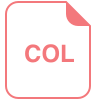
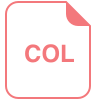
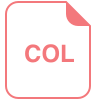









