springboot自定义starter示例
时间: 2023-06-28 18:16:22 浏览: 133
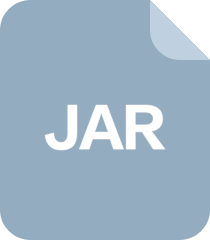
spring-boot自定义starter的demo
下面是一个简单的Spring Boot自定义Starter的示例,该Starter实现了一个自定义的HelloWorld功能:
1. 创建Maven项目
首先,我们需要创建一个Maven项目作为我们自定义Starter的项目。在项目的pom.xml中添加Spring Boot的依赖,以及其他需要集成的依赖。
2. 编写自动配置类
在src/main/java目录下创建一个名为HelloWorldAutoConfiguration的类,该类用于自动配置HelloWorld功能:
```java
@Configuration
@ConditionalOnClass(HelloWorldService.class)
@EnableConfigurationProperties(HelloWorldProperties.class)
public class HelloWorldAutoConfiguration {
@Autowired
private HelloWorldProperties properties;
@Bean
@ConditionalOnMissingBean
public HelloWorldService helloWorldService() {
HelloWorldService service = new HelloWorldService();
service.setMsg(properties.getMsg());
return service;
}
@ConfigurationProperties(prefix = "hello.world")
public static class HelloWorldProperties {
private String msg = "Hello, world!";
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
}
```
上述代码中,@Configuration注解表示该类是一个配置类,@ConditionalOnClass注解表示只有当HelloWorldService类存在时才进行配置,@EnableConfigurationProperties注解表示将HelloWorldProperties类注入到Spring容器中。在helloWorldService方法中,我们通过读取HelloWorldProperties中的配置来创建一个HelloWorldService实例。
3. 编写Starter类
在src/main/java目录下创建一个名为HelloWorldStarter的类,该类用于将自动配置类注入到Spring容器中:
```java
@Configuration
@EnableConfigurationProperties(HelloWorldProperties.class)
@Import(HelloWorldAutoConfiguration.class)
public class HelloWorldStarter {
}
```
上述代码中,@Configuration注解表示该类是一个配置类,@EnableConfigurationProperties注解表示将HelloWorldProperties类注入到Spring容器中,@Import注解表示将HelloWorldAutoConfiguration类注入到Spring容器中。
4. 打包和发布Starter
在命令行中运行以下命令,将自定义Starter打包成jar包:
```
mvn clean package
```
然后将jar包发布到Maven仓库中。
5. 在项目中使用自定义Starter
在其他Spring Boot项目中的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.example</groupId>
<artifactId>hello-world-starter</artifactId>
<version>1.0.0</version>
</dependency>
```
在项目中使用以下代码来测试自定义Starter是否生效:
```java
@RestController
public class TestController {
@Autowired
private HelloWorldService helloWorldService;
@GetMapping("/hello")
public String hello() {
return helloWorldService.sayHello();
}
}
```
上述代码中,我们通过@Autowired注解注入了HelloWorldService实例,并在hello方法中调用了sayHello方法来测试自定义Starter是否生效。
以上就是Spring Boot自定义Starter的一个简单示例,通过自定义Starter,我们可以将自己的功能快速集成到Spring Boot中,提高开发效率。
阅读全文
相关推荐
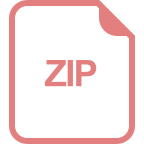
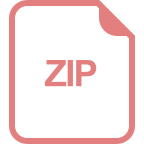
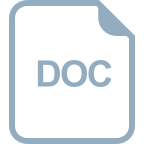
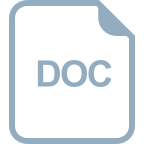
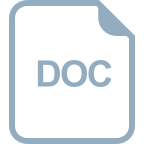

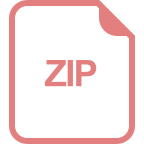
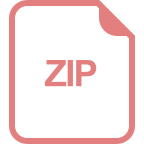
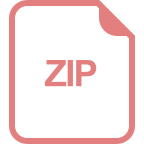
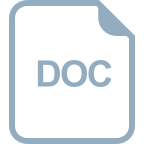



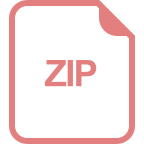
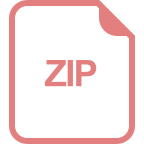
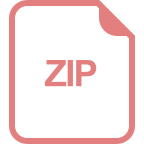