写一个python俄罗斯方块
时间: 2023-07-25 22:06:32 浏览: 46
好的,下面是一个基本的 Python 俄罗斯方块游戏实现:
```python
import pygame
import random
# 定义方块大小和游戏区域大小
BLOCK_SIZE = 20
GAME_AREA_WIDTH = 10 * BLOCK_SIZE
GAME_AREA_HEIGHT = 20 * BLOCK_SIZE
# 定义方块类型和对应的形状
BLOCK_TYPES = [
{
'shape': [[1, 1], [1, 1]],
'color': (255, 0, 0)
},
{
'shape': [[0, 1, 0], [1, 1, 1]],
'color': (0, 255, 0)
},
{
'shape': [[1, 1, 0], [0, 1, 1]],
'color': (0, 0, 255)
},
{
'shape': [[0, 1, 1], [1, 1, 0]],
'color': (255, 255, 0)
},
{
'shape': [[1, 0, 0], [1, 1, 1]],
'color': (255, 0, 255)
},
{
'shape': [[0, 0, 1], [1, 1, 1]],
'color': (0, 255, 255)
},
{
'shape': [[1, 1, 1, 1]],
'color': (128, 128, 128)
}
]
class Block:
def __init__(self, x, y, shape, color):
self.x = x
self.y = y
self.shape = shape
self.color = color
def rotate(self):
"""旋转方块"""
self.shape = [[self.shape[j][i] for j in range(len(self.shape))] for i in range(len(self.shape[0])-1, -1, -1)]
def move_left(self):
"""左移方块"""
self.x -= BLOCK_SIZE
def move_right(self):
"""右移方块"""
self.x += BLOCK_SIZE
def move_down(self):
"""下移方块"""
self.y += BLOCK_SIZE
class Game:
def __init__(self):
pygame.init()
# 设置游戏窗口和标题
self.screen = pygame.display.set_mode((GAME_AREA_WIDTH + 200, GAME_AREA_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 加载字体
self.font = pygame.font.SysFont(None, 48)
# 随机生成方块
self.current_block = None
self.next_block = self._generate_block()
# 游戏区域
self.game_area = [[None for _ in range(10)] for _ in range(20)]
# 初始化游戏状态
self.score = 0
self.game_over = False
# 游戏循环
self.clock = pygame.time.Clock()
self.fps = 5
def _generate_block(self):
"""随机生成方块"""
block_type = random.choice(BLOCK_TYPES)
shape = block_type['shape']
color = block_type['color']
x = GAME_AREA_WIDTH // 2 - len(shape[0]) * BLOCK_SIZE // 2
y = -len(shape) * BLOCK_SIZE
return Block(x, y, shape, color)
def _draw_block(self, block):
"""绘制方块"""
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j] == 1:
rect = pygame.Rect(block.x + j * BLOCK_SIZE, block.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(self.screen, block.color, rect)
def _draw_game_area(self):
"""绘制游戏区域"""
for i in range(len(self.game_area)):
for j in range(len(self.game_area[0])):
if self.game_area[i][j]:
rect = pygame.Rect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(self.screen, self.game_area[i][j], rect)
def _check_collision(self, block):
"""检查方块是否碰撞"""
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j] == 1:
x, y = block.x + j * BLOCK_SIZE, block.y + i * BLOCK_SIZE
if x < 0 or x >= GAME_AREA_WIDTH or y >= GAME_AREA_HEIGHT:
return True
if y >= 0 and self.game_area[y//BLOCK_SIZE][x//BLOCK_SIZE]:
return True
return False
def _add_block_to_game_area(self, block):
"""将方块加入游戏区域"""
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j] == 1:
x, y = block.x + j * BLOCK_SIZE, block.y + i * BLOCK_SIZE
if y >= 0:
self.game_area[y//BLOCK_SIZE][x//BLOCK_SIZE] = block.color
def _check_full_lines(self):
"""检查是否有满行"""
full_lines = []
for i in range(len(self.game_area)):
if all(self.game_area[i]):
full_lines.append(i)
return full_lines
def _remove_full_lines(self, full_lines):
"""移除满行"""
for i in full_lines:
del self.game_area[i]
self.game_area.insert(0, [None for _ in range(10)])
def _update_score(self, full_lines):
"""更新得分"""
self.score += len(full_lines) * 100
def _draw_score(self):
"""绘制得分"""
score_text = self.font.render(f"Score: {self.score}", True, (255, 255, 255))
self.screen.blit(score_text, (GAME_AREA_WIDTH + 20, 20))
def _draw_game_over(self):
"""绘制游戏结束"""
game_over_text = self.font.render("Game Over", True, (255, 0, 0))
self.screen.blit(game_over_text, (GAME_AREA_WIDTH // 2 - game_over_text.get_width() // 2, GAME_AREA_HEIGHT // 2 - game_over_text.get_height() // 2))
def run(self):
"""游戏主循环"""
while not self.game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and self.current_block:
self.current_block.move_left()
if self._check_collision(self.current_block):
self.current_block.move_right()
elif event.key == pygame.K_RIGHT and self.current_block:
self.current_block.move_right()
if self._check_collision(self.current_block):
self.current_block.move_left()
elif event.key == pygame.K_DOWN and self.current_block:
self.current_block.move_down()
if self._check_collision(self.current_block):
self.current_block.move_up()
elif event.key == pygame.K_UP and self.current_block:
self.current_block.rotate()
if self._check_collision(self.current_block):
self.current_block.rotate()
# 更新方块位置
if not self.current_block:
self.current_block = self.next_block
self.next_block = self._generate_block()
if self._check_collision(self.current_block):
self.game_over = True
else:
self.current_block.move_down()
if self._check_collision(self.current_block):
self.current_block.move_up()
self._add_block_to_game_area(self.current_block)
full_lines = self._check_full_lines()
if full_lines:
self._remove_full_lines(full_lines)
self._update_score(full_lines)
self.current_block = None
# 绘制游戏界面
self.screen.fill((0, 0, 0))
self._draw_game_area()
self._draw_block(self.next_block)
if self.current_block:
self._draw_block(self.current_block)
self._draw_score()
if self.game_over:
self._draw_game_over()
pygame.display.flip()
# 控制帧率
self.clock.tick(self.fps)
pygame.quit()
if __name__ == '__main__':
game = Game()
game.run()
```
这个程序使用 Pygame 库实现,可以通过左右方向键移动方块,上方向键旋转方块,下方向键加速方块下落。游戏结束后会显示得分和 "Game Over" 的字样。
相关推荐
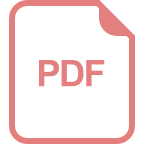
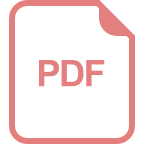
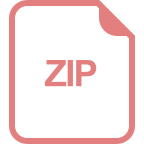
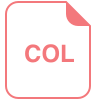
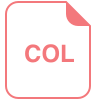
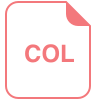
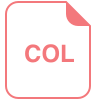
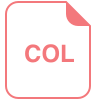








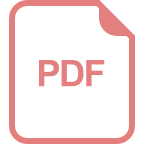