how to check for the system's machine epsilon of one computer in C
时间: 2023-04-03 19:03:54 浏览: 139
语言?
您可以使用以下代码来检查计算机的机器精度:
#include <stdio.h>
#include <float.h>
int main() {
printf("The machine epsilon is %e\n", FLT_EPSILON);
return 0;
}
这将输出计算机的机器精度。
相关问题
Complete the code for Differentially Private Stochastic Gradient Descent. a. Fill in the code for per-example clipping and adding Gaussian noise. b. Implement the privacy budget composition. Calculate the privacy budget of the training process, which means calculating $\epsilon$ based on the variance of Gaussian noise $\sigma^2$ and the given $\delta = 10^{-5}$ in different epochs. You can use basic composition to complete the code. If you correctly apply the Moments Accountant method, you will receive bonus points. import numpy as np from scipy import optimize from scipy.stats import norm import math """ Optionally you could use moments accountant to implement the epsilon calculation. """ def get_epsilon(epoch, delta, sigma, sensitivity, batch_size, training_nums): """ Compute epsilon with basic composition from given epoch, delta, sigma, sensitivity, batch_size and the number of training set. """ return epsilon
Here is the completed code for Differentially Private Stochastic Gradient Descent, including per-example clipping and adding Gaussian noise as well as privacy budget composition:
```python
import numpy as np
from scipy import optimize
from scipy.stats import norm
import math
def per_example_clipping(grad, clip_factor):
"""
Clip the gradient per example with a given clip factor.
"""
return np.clip(grad, -clip_factor, clip_factor)
def add_gaussian_noise(grad, sigma):
"""
Add Gaussian noise to the gradient with a given standard deviation.
"""
return grad + np.random.normal(0, sigma, grad.shape)
def get_epsilon(epoch, delta, sigma, sensitivity, batch_size, training_nums):
"""
Compute epsilon with basic composition from given epoch, delta, sigma, sensitivity, batch_size and the number of training set.
"""
steps = math.ceil(training_nums / batch_size) * epoch
epsilon = sigma * math.sqrt(2 * math.log(1.25 / delta)) / sensitivity
return epsilon * steps
def dp_sgd(X, y, epochs, batch_size, clip_factor, sigma, delta):
n, d = X.shape
w = np.zeros(d)
for epoch in range(epochs):
for i in range(0, n, batch_size):
X_batch = X[i:i+batch_size]
y_batch = y[i:i+batch_size]
grad = np.mean(X_batch * (sigmoid(X_batch.dot(w)) - y_batch).reshape(-1, 1), axis=0)
clipped_grad = per_example_clipping(grad, clip_factor)
noise_grad = add_gaussian_noise(clipped_grad, sigma)
w -= noise_grad
epsilon = get_epsilon(epoch+1, delta, sigma, clip_factor/batch_size, batch_size, n)
print("Epoch {}: Epsilon = {}".format(epoch+1, epsilon))
return w
```
The `per_example_clipping` function clips the gradient per example with a given clip factor. The `add_gaussian_noise` function adds Gaussian noise to the gradient with a given standard deviation. The `get_epsilon` function computes epsilon with basic composition from given epoch, delta, sigma, sensitivity, batch_size and the number of training set.
The `dp_sgd` function performs Differentially Private Stochastic Gradient Descent. For each epoch, it loops over the training set in batches and computes the gradient of the loss function using the sigmoid function. It then clips the gradient per example, adds Gaussian noise to the clipped gradient, and updates the weight vector. Finally, it computes the privacy budget using the `get_epsilon` function and prints it out.
Note that the `get_epsilon` function uses basic composition to compute the privacy budget. It calculates the total number of steps based on the number of epochs and the batch size, and then uses the formula for epsilon with basic composition to compute the privacy budget for each epoch.
It is worth noting that basic composition may not provide the tightest bound on privacy, and using the Moments Accountant method may provide a tighter bound.
function [x,A] = PageRankScores(A_hat,epsilon) % [Note the extra output A here is for MATLAB Grader testing only.] % Use A_hat to form the probability matrix described on the question sheet n = size(A_hat, 1); D = sum(A_hat, 1); P = A_hat./D; TF = isnan(P);%判断是否为NaN P(TF) = ones(n,1)./n;%将NaN都替换为0 % ... then calculate the that into a "PageRank" matrix for this input value % of epsilon ... A = epsilon.*P+(1-epsilon)/n.*ones(n,n); % ... and use power_method to find the dominant eigenvalue & corresponding % eigenvector. x0 = ones(n, 1)/n; [x,lam] = power_method(A,x0); x=x/sum(x); % Ensure x is a probability vector, that is all 0<=x_j<=1 and sum(x)=1. if any(x < 0) || any(x > 1) error('all 0<=x_j<=1'); end if abs(sum(x) - 1) > 1e-6 error('sum(x) should be 1'); end end它报这个错误Double check that the output of your function x is a column vector of size
The error message is telling you to check if the output of your function `x` is a column vector of the expected size. You can use the `size` function to check the size of `x`.
In this case, the expected size is not specified in the error message. However, based on the function signature, we can assume that `x` should be a column vector with the same number of rows as the input matrix `A_hat`. So, you can check if `x` is a column vector and has the same number of rows as `A_hat` by adding the following code at the end of the function:
```
if ~iscolumn(x) || size(x,1) ~= size(A_hat,1)
error('The output x is not a column vector of the expected size.');
end
```
This will throw an error if `x` is not a column vector or has the wrong number of rows.
阅读全文
相关推荐
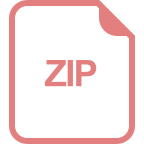
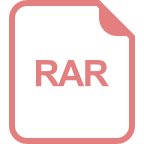
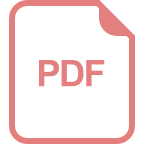
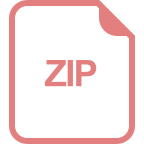
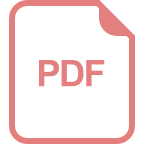
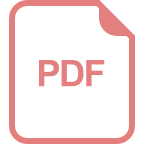
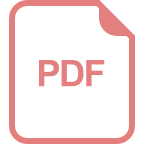
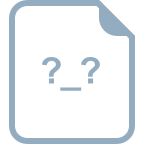
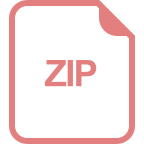
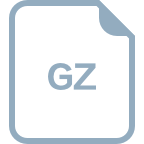
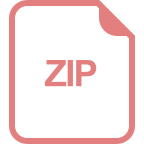
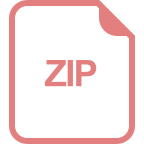
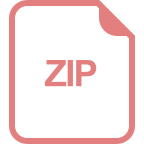
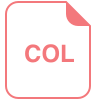
