cohen-sutherland算法c++
时间: 2023-04-26 16:04:50 浏览: 177
Cohen-Sutherland算法是一种用于计算机图形学中的线段裁剪算法。它是由Nicholas Cohen和Ivan Sutherland在1967年提出的。该算法可以快速地确定线段是否在裁剪窗口内,并将其裁剪为仅在窗口内的线段。
Cohen-Sutherland算法的C++实现通常包括以下步骤:
1. 定义裁剪窗口的边界,通常是一个矩形。
2. 将线段的两个端点分别标记为P1和P2。
3. 对于每个端点,计算其区域码。区域码是一个4位二进制数,用于表示该点的位置关系。例如,如果点在裁剪窗口的左边,则其区域码的第一位为1。
4. 检查P1和P2的区域码,如果它们都为0,则线段完全在裁剪窗口内,不需要进行裁剪。
5. 如果P1和P2的区域码都不为0,则线段可能与裁剪窗口相交。使用区域码的逻辑运算来确定线段与裁剪窗口的交点。
6. 如果线段与裁剪窗口相交,则将其裁剪为仅在窗口内的线段。
7. 如果线段与裁剪窗口不相交,则将其舍弃。
C++实现中,可以使用结构体来表示点和线段,使用位运算来计算区域码,使用if语句和逻辑运算来实现裁剪。
相关问题
cohen-sutherland算法c++代码
以下是C语言实现的Cohen-Sutherland算法代码:
#include <stdio.h>
#include <graphics.h>
#define INSIDE 0
#define LEFT 1
#define RIGHT 2
#define BOTTOM 4
#define TOP 8
int xmin, ymin, xmax, ymax;
int ComputeOutCode(int x, int y) {
int code = INSIDE;
if (x < xmin) {
code |= LEFT;
} else if (x > xmax) {
code |= RIGHT;
}
if (y < ymin) {
code |= BOTTOM;
} else if (y > ymax) {
code |= TOP;
}
return code;
}
void CohenSutherland(int x1, int y1, int x2, int y2) {
int outcode1 = ComputeOutCode(x1, y1);
int outcode2 = ComputeOutCode(x2, y2);
while (1) {
if (!(outcode1 | outcode2)) {
// Both points inside window
line(x1, y1, x2, y2);
break;
} else if (outcode1 & outcode2) {
// Both points outside window and same region
break;
} else {
int x, y, outcode;
// At least one endpoint is outside the window
if (outcode1) {
outcode = outcode1;
} else {
outcode = outcode2;
}
// Find intersection point
if (outcode & TOP) {
x = x1 + (x2 - x1) * (ymax - y1) / (y2 - y1);
y = ymax;
} else if (outcode & BOTTOM) {
x = x1 + (x2 - x1) * (ymin - y1) / (y2 - y1);
y = ymin;
} else if (outcode & RIGHT) {
y = y1 + (y2 - y1) * (xmax - x1) / (x2 - x1);
x = xmax;
} else if (outcode & LEFT) {
y = y1 + (y2 - y1) * (xmin - x1) / (x2 - x1);
x = xmin;
}
// Update the point and outcode
if (outcode == outcode1) {
x1 = x;
y1 = y;
outcode1 = ComputeOutCode(x1, y1);
} else {
x2 = x;
y2 = y;
outcode2 = ComputeOutCode(x2, y2);
}
}
}
}
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
xmin = 50;
ymin = 50;
xmax = 200;
ymax = 200;
rectangle(xmin, ymin, xmax, ymax);
// Test cases
CohenSutherland(10, 10, 300, 300);
CohenSutherland(0, 150, 250, 150);
CohenSutherland(150, 0, 150, 250);
CohenSutherland(250, 250, 350, 350);
getch();
closegraph();
return 0;
}
cpp代码实现直线裁剪算法Cohen-Sutherland算法
Cohen-Sutherland算法是一种经典的二维图形变换算法,用于判断交线、裁剪或忽略两个已知边界线段之间的交叉。在C++中,可以使用以下伪代码表示基本的Cohen-Sutherland算法:
```cpp
enum class EdgeStatus { OUTSIDE, INTERSECT, INSIDE };
EdgeStatus clipLine(int ax, int ay, int bx, int by, int cx, int cy, int dx, int dy) {
// 边界条件:两条线平行或者相交
if (bx - ax == 0 && by - ay == 0) return EdgeStatus::INTERSECT; // 平行
if (dx - cx == 0 && dy - cy == 0) return EdgeStatus::INTERSECT; // 平行
// 定义四个方向的斜率(当直线不垂直时)
double slope1 = (by - ay) / (bx - ax);
double slope2 = (dy - cy) / (dx - cx);
// 计算当前线段的交点坐标(如果存在交点)
double u1 = (cy - ay) * slope1 - cx + ax;
double u2 = (cy - cy) * slope2 - cx + dx;
// 判断当前线段与屏幕边界的关系
if (u1 > 0 && u1 < 1) return EdgeStatus::INSIDE;
if (u2 > 0 && u2 < 1) return EdgeStatus::INSIDE;
// 线段与屏幕边界垂直的情况
if (slope1 != slope2) {
if (slope1 == INFINITY || slope2 == INFINITY) return EdgeStatus::OUTSIDE;
double v1 = (ax - cx) / slope1;
double v2 = (cx - dx) / slope2;
if (v1 > 0 && v1 < 1) return EdgeStatus::INSIDE;
if (v2 > 0 && v2 < 1) return EdgeStatus::INSIDE;
}
// 如果都未命中边界,则线段与屏幕完全外接
return EdgeStatus::OUTSIDE;
}
// 示例:对屏幕(0,0)至(width,height)的矩形进行裁剪
std::pair<bool, int> applyClip(int ax, int ay, int bx, int by, int width, int height) {
bool inside = false;
int result = EdgeStatus::OUTSIDE;
// 裁剪左边界
result = clipLine(ax, ay, bx, by, 0, 0, width, 0);
inside |= result == EdgeStatus::INSIDE;
// 裁剪上边界
result = clipLine(ax, ay, bx, by, 0, 0, 0, height);
inside |= result == EdgeStatus::INSIDE;
// 裁剪右边界
result = clipLine(ax, ay, bx, by, width, height, width, 0);
inside |= result == EdgeStatus::INSIDE;
// 裁剪下边界
result = clipLine(ax, ay, bx, by, 0, height, 0, height);
inside |= result == EdgeStatus::INSIDE;
return {inside, result};
}
// 使用示例
std::pair<bool, int> clippedLine = applyClip(10, 10, 20, 20, 400, 400);
if (clippedLine.first)
std::cout << "Line segment is visible on screen.\n";
else
std::cout << "Line segment is clipped or outside the screen.\n";
```
阅读全文
相关推荐
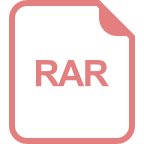








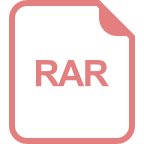



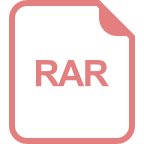