请给出c++实现cohen-sutherland裁剪算法的具体代码
时间: 2023-06-11 09:04:52 浏览: 134
以下是一个简单的C语言实现cohen-sutherland裁剪算法的代码:
```c
#include<stdio.h>
int INSIDE = 0;
int LEFT = 1;
int RIGHT = 2;
int BOTTOM = 4;
int TOP = 8;
double x_max, y_max, x_min, y_min;
int getCode(double x, double y) {
int code = INSIDE;
if(x < x_min)
code |= LEFT;
else if(x > x_max)
code |= RIGHT;
if(y < y_min)
code |= BOTTOM;
else if(y > y_max)
code |= TOP;
return code;
}
void cohenSutherlandClip(double x1, double y1, double x2, double y2) {
int code1 = getCode(x1, y1);
int code2 = getCode(x2, y2);
int accept = 0;
while(1) {
if((code1 == 0) && (code2 == 0)) { //Both points inside
accept = 1;
break;
} else if (code1 & code2) { //Both points outside, and in same region
break;
} else {
int code_out;
double x, y;
if(code1 != 0) {
code_out = code1;
} else {
code_out = code2;
}
if (code_out & TOP) {
x = x1 + (x2 - x1) * (y_max - y1) / (y2 - y1);
y = y_max;
} else if(code_out & BOTTOM) {
x = x1 + (x2 - x1) * (y_min - y1) / (y2 - y1);
y = y_min;
} else if(code_out & RIGHT) {
y = y1 + (y2 - y1) * (x_max - x1) / (x2 - x1);
x = x_max;
} else if(code_out & LEFT) {
y = y1 + (y2 - y1) * (x_min - x1) / (x2 - x1);
x = x_min;
}
if(code_out == code1) {
x1 = x; y1 = y;
code1 = getCode(x1, y1);
} else {
x2 = x; y2 = y;
code2 = getCode(x2, y2);
}
}
}
if(accept) {
printf("Line accepted from (%lf,%lf) to (%lf,%lf)\n", x1, y1, x2, y2);
} else {
printf("Line rejected\n");
}
}
int main() {
printf("Enter x_min: ");
scanf("%lf", &x_min);
printf("Enter y_min: ");
scanf("%lf", &y_min);
printf("Enter x_max: ");
scanf("%lf", &x_max);
printf("Enter y_max: ");
scanf("%lf", &y_max);
double x1, y1, x2, y2;
printf("Enter x1: ");
scanf("%lf", &x1);
printf("Enter y1: ");
scanf("%lf", &y1);
printf("Enter x2: ");
scanf("%lf", &x2);
printf("Enter y2: ");
scanf("%lf", &y2);
cohenSutherlandClip(x1, y1, x2, y2);
return 0;
}
```
这是一个控制台程序,在运行时会提示用户输入裁剪区域的坐标和待裁剪的线段的坐标,然后输出裁剪后的结果或拒绝裁剪的信息。
阅读全文
相关推荐



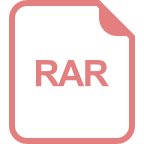


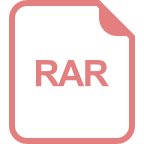







