cpp代码实现直线裁剪算法Cohen-Sutherland算法
时间: 2024-10-18 08:16:26 浏览: 80
Cohen-Sutherland算法是一种经典的二维图形变换算法,用于判断交线、裁剪或忽略两个已知边界线段之间的交叉。在C++中,可以使用以下伪代码表示基本的Cohen-Sutherland算法:
```cpp
enum class EdgeStatus { OUTSIDE, INTERSECT, INSIDE };
EdgeStatus clipLine(int ax, int ay, int bx, int by, int cx, int cy, int dx, int dy) {
// 边界条件:两条线平行或者相交
if (bx - ax == 0 && by - ay == 0) return EdgeStatus::INTERSECT; // 平行
if (dx - cx == 0 && dy - cy == 0) return EdgeStatus::INTERSECT; // 平行
// 定义四个方向的斜率(当直线不垂直时)
double slope1 = (by - ay) / (bx - ax);
double slope2 = (dy - cy) / (dx - cx);
// 计算当前线段的交点坐标(如果存在交点)
double u1 = (cy - ay) * slope1 - cx + ax;
double u2 = (cy - cy) * slope2 - cx + dx;
// 判断当前线段与屏幕边界的关系
if (u1 > 0 && u1 < 1) return EdgeStatus::INSIDE;
if (u2 > 0 && u2 < 1) return EdgeStatus::INSIDE;
// 线段与屏幕边界垂直的情况
if (slope1 != slope2) {
if (slope1 == INFINITY || slope2 == INFINITY) return EdgeStatus::OUTSIDE;
double v1 = (ax - cx) / slope1;
double v2 = (cx - dx) / slope2;
if (v1 > 0 && v1 < 1) return EdgeStatus::INSIDE;
if (v2 > 0 && v2 < 1) return EdgeStatus::INSIDE;
}
// 如果都未命中边界,则线段与屏幕完全外接
return EdgeStatus::OUTSIDE;
}
// 示例:对屏幕(0,0)至(width,height)的矩形进行裁剪
std::pair<bool, int> applyClip(int ax, int ay, int bx, int by, int width, int height) {
bool inside = false;
int result = EdgeStatus::OUTSIDE;
// 裁剪左边界
result = clipLine(ax, ay, bx, by, 0, 0, width, 0);
inside |= result == EdgeStatus::INSIDE;
// 裁剪上边界
result = clipLine(ax, ay, bx, by, 0, 0, 0, height);
inside |= result == EdgeStatus::INSIDE;
// 裁剪右边界
result = clipLine(ax, ay, bx, by, width, height, width, 0);
inside |= result == EdgeStatus::INSIDE;
// 裁剪下边界
result = clipLine(ax, ay, bx, by, 0, height, 0, height);
inside |= result == EdgeStatus::INSIDE;
return {inside, result};
}
// 使用示例
std::pair<bool, int> clippedLine = applyClip(10, 10, 20, 20, 400, 400);
if (clippedLine.first)
std::cout << "Line segment is visible on screen.\n";
else
std::cout << "Line segment is clipped or outside the screen.\n";
```
阅读全文
相关推荐
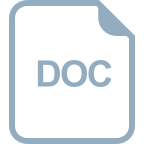
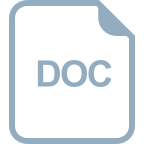
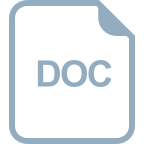
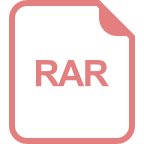
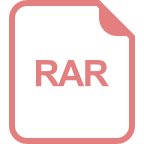
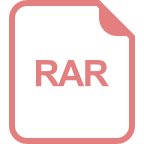
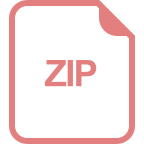
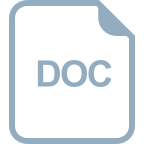


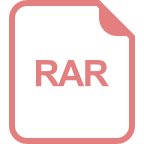
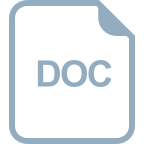
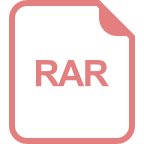
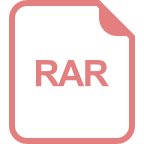
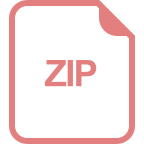
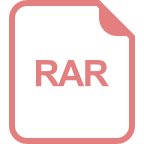
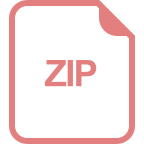
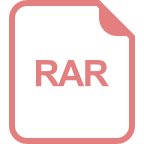