java 3d旋转图片_实例演示javafx实现图片3D翻转的效果
时间: 2023-07-15 17:10:38 浏览: 48
下面是一个简单的 JavaFX 实现图片 3D 翻转效果的示例代码:
```java
import javafx.animation.Interpolator;
import javafx.animation.KeyFrame;
import javafx.animation.KeyValue;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.geometry.Point3D;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.media.AudioClip;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Rotate;
import javafx.stage.Stage;
import javafx.util.Duration;
public class Image3DFlipDemo extends Application {
private static final String AUDIO_URL = "http://www.music.helsinki.fi/tmt/opetus/uusmedia/esim/a2002011001-e02.wav";
private static final double IMAGE_WIDTH = 400.0;
private static final double IMAGE_HEIGHT = 300.0;
private ImageView imageView;
private AudioClip audioClip;
@Override
public void start(Stage stage) throws Exception {
// 创建 ImageView 并加载图片
Image image = new Image("https://picsum.photos/" + (int) IMAGE_WIDTH + "/" + (int) IMAGE_HEIGHT);
imageView = new ImageView(image);
imageView.setFitWidth(IMAGE_WIDTH);
imageView.setFitHeight(IMAGE_HEIGHT);
// 创建背景矩形
Rectangle bgRect = new Rectangle(IMAGE_WIDTH, IMAGE_HEIGHT, Color.BLACK);
// 创建容器 Group 并添加 ImageView 和背景矩形
Group group = new Group(bgRect, imageView);
// 创建场景 Scene 并添加容器 Group
Scene scene = new Scene(group, IMAGE_WIDTH, IMAGE_HEIGHT, Color.BLACK);
// 创建旋转变换 Rotate
Rotate rotate = new Rotate(0, new Point3D(0, 0, 0));
// 将旋转变换 Rotate 添加到 ImageView 上
imageView.getTransforms().add(rotate);
// 创建 Timeline 并添加旋转动画
Timeline timeline = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(rotate.angleProperty(), 0, Interpolator.LINEAR)),
new KeyFrame(Duration.seconds(1), new KeyValue(rotate.angleProperty(), 180, Interpolator.EASE_OUT))
);
// 设置 Timeline 循环次数为 2
timeline.setCycleCount(2);
// 设置 Timeline 播放完成后的回调函数
timeline.setOnFinished(event -> {
// 将 ImageView 的图片翻转为镜像
imageView.setScaleX(imageView.getScaleX() * -1);
// 播放音效
if (audioClip != null) {
audioClip.play();
}
});
// 设置场景 Scene 的鼠标点击事件
scene.setOnMouseClicked(event -> {
// 如果 Timeline 正在播放,则返回
if (timeline.getStatus() == Timeline.Status.RUNNING) {
return;
}
// 设置 Timeline 的起始值为当前旋转角度
double startAngle = rotate.getAngle();
// 创建 KeyValue 并添加到 Timeline 中
KeyValue keyValue = new KeyValue(rotate.angleProperty(), startAngle + 180, Interpolator.EASE_IN);
timeline.getKeyFrames().set(1, new KeyFrame(Duration.seconds(1), keyValue));
// 播放 Timeline
timeline.play();
});
// 创建音效 AudioClip
audioClip = new AudioClip(AUDIO_URL);
// 显示场景 Scene
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在上面的代码中,我们创建了一个 ImageView 并加载了一张图片,然后创建了一个背景矩形和一个容器 Group,并将 ImageView 和背景矩形添加到容器 Group 中,最后创建了一个场景 Scene 并将容器 Group 添加到场景 Scene 中。
接着,我们创建了一个旋转变换 Rotate,并将其添加到 ImageView 上,然后创建了一个 Timeline 并添加了旋转动画,然后设置 Timeline 循环次数为 2,并设置 Timeline 播放完成后的回调函数,回调函数中将 ImageView 的图片翻转为镜像,并播放了一个音效。
最后,我们设置了场景 Scene 的鼠标点击事件,当鼠标点击场景时,如果 Timeline 正在播放,则直接返回,否则设置 Timeline 的起始值为当前旋转角度,并播放 Timeline。
你可以将上面的代码复制到一个 Java 类中并运行,然后点击场景看看图片的 3D 翻转效果。
相关推荐
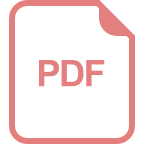














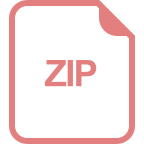