用c语言建立一个二叉链表
时间: 2024-03-23 09:39:03 浏览: 55
好的,以下是用C语言建立二叉链表的基本步骤:
首先,我们需要定义二叉树结点的结构体:
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
```
接着,我们可以编写一个函数来创建一个新的结点:
```c
struct TreeNode* createNode(int val) {
struct TreeNode* newNode = (struct TreeNode*)malloc(sizeof(struct TreeNode));
newNode->val = val;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
```
然后,我们可以编写一个函数来插入一个新的结点到二叉树中:
```c
void insert(struct TreeNode** root, int val) {
if (*root == NULL) {
*root = createNode(val);
return;
}
if (val < (*root)->val) {
insert(&((*root)->left), val);
} else {
insert(&((*root)->right), val);
}
}
```
最后,我们可以编写一个函数来遍历整个二叉树:
```c
void traverse(struct TreeNode* root) {
if (root == NULL) {
return;
}
traverse(root->left);
printf("%d ", root->val);
traverse(root->right);
}
```
这些函数可以组合在一起来构建一个完整的二叉链表程序,示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
struct TreeNode* createNode(int val) {
struct TreeNode* newNode = (struct TreeNode*)malloc(sizeof(struct TreeNode));
newNode->val = val;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
void insert(struct TreeNode** root, int val) {
if (*root == NULL) {
*root = createNode(val);
return;
}
if (val < (*root)->val) {
insert(&((*root)->left), val);
} else {
insert(&((*root)->right), val);
}
}
void traverse(struct TreeNode* root) {
if (root == NULL) {
return;
}
traverse(root->left);
printf("%d ", root->val);
traverse(root->right);
}
int main() {
struct TreeNode* root = NULL;
insert(&root, 5);
insert(&root, 3);
insert(&root, 7);
insert(&root, 1);
insert(&root, 4);
insert(&root, 6);
insert(&root, 8);
traverse(root);
return 0;
}
```
输出结果为:1 3 4 5 6 7 8
阅读全文
相关推荐
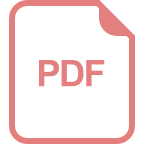






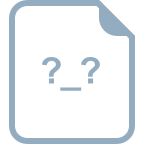
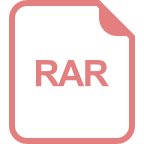
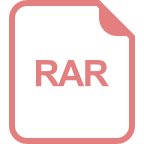
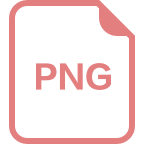