我觉得您可以帮我写一个五子棋游戏
时间: 2023-07-01 08:13:47 浏览: 49
好的,那我可以为您提供一个 Python 实现的五子棋游戏的框架代码,您可以在此基础上进行修改和完善。以下是代码:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 创建游戏窗口
screen_width, screen_height = 600, 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("五子棋")
# 定义棋盘尺寸和格子大小
board_size = 15
grid_size = screen_width // board_size
# 定义棋盘数组
board = [[0] * board_size for _ in range(board_size)]
# 定义当前玩家,1 表示黑子,2 表示白子
current_player = 1
# 绘制棋盘
def draw_board():
# 绘制棋盘背景
screen.fill((255, 206, 158))
# 绘制棋盘网格
for i in range(board_size):
pygame.draw.line(screen, (0, 0, 0), (grid_size, (i + 1) * grid_size), (screen_width - grid_size, (i + 1) * grid_size))
pygame.draw.line(screen, (0, 0, 0), ((i + 1) * grid_size, grid_size), ((i + 1) * grid_size, screen_height - grid_size))
# 绘制棋子
for i in range(board_size):
for j in range(board_size):
if board[i][j] == 1:
pygame.draw.circle(screen, (0, 0, 0), ((j + 1) * grid_size, (i + 1) * grid_size), grid_size // 2 - 2)
elif board[i][j] == 2:
pygame.draw.circle(screen, (255, 255, 255), ((j + 1) * grid_size, (i + 1) * grid_size), grid_size // 2 - 2)
# 落子函数
def place_piece(row, col, player):
board[row][col] = player
# 判断胜负函数
def check_win(player):
# 判断横向是否有五子连珠
for i in range(board_size):
for j in range(board_size - 4):
if board[i][j] == player and board[i][j + 1] == player and board[i][j + 2] == player and board[i][j + 3] == player and board[i][j + 4] == player:
return True
# 判断竖向是否有五子连珠
for i in range(board_size - 4):
for j in range(board_size):
if board[i][j] == player and board[i + 1][j] == player and board[i + 2][j] == player and board[i + 3][j] == player and board[i + 4][j] == player:
return True
# 判断左上到右下斜向是否有五子连珠
for i in range(board_size - 4):
for j in range(board_size - 4):
if board[i][j] == player and board[i + 1][j + 1] == player and board[i + 2][j + 2] == player and board[i + 3][j + 3] == player and board[i + 4][j + 4] == player:
return True
# 判断左下到右上斜向是否有五子连珠
for i in range(4, board_size):
for j in range(board_size - 4):
if board[i][j] == player and board[i - 1][j + 1] == player and board[i - 2][j + 2] == player and board[i - 3][j + 3] == player and board[i - 4][j + 4] == player:
return True
return False
# 主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
# 获取鼠标点击位置
mouse_x, mouse_y = event.pos
# 计算行列数
row, col = mouse_y // grid_size - 1, mouse_x // grid_size - 1
# 判断是否越界
if row < 0 or row >= board_size or col < 0 or col >= board_size:
continue
# 判断该位置是否已经有棋子
if board[row][col] != 0:
continue
# 落子
place_piece(row, col, current_player)
# 判断胜负
if check_win(current_player):
print("Player %d wins!" % current_player)
running = False
# 切换玩家
current_player = 2 if current_player == 1 else 1
# 绘制游戏界面
draw_board()
# 更新游戏窗口
pygame.display.update()
# 退出 Pygame
pygame.quit()
```
这份代码使用 Pygame 图形库实现五子棋游戏的基本功能,包括绘制棋盘、落子、判断胜负等。您可以根据自己的需求和实际情况进行修改和完善。希望这份代码对您有所帮助。
相关推荐
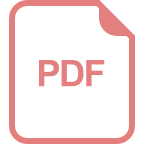
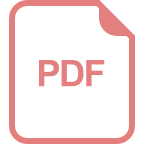
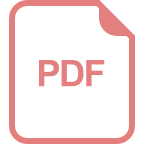














