用java来实现利用文件构建用户电话号码本。(需实现增、删、查(按指定属性排序并查询)、改、显示的功能)。 1. “添加”功能(addEntry):接收用户的输入,将新的记录添加到文件中 2. “删除”功能(removeEntry):接收用户输入的姓名或电话号码,搜索文件中相应的记录并将其删除。 3. “查询”功能(sortedSearch):接收用户输入的排序方式和查询关键词,对文件中的记录按照指定属性进行排序并进行查询。 4. “修改”功能(updateEntry):接收用户输入的姓名或电话号码,搜索文件中相应的记录并将其更新为新输入的信息。 5. “显示”功能(displayEntries):从文件中读取并展示所有已存储的记录。
时间: 2024-02-01 22:14:53 浏览: 22
以下是一个简单的示例代码:
```java
import java.io.*;
import java.util.*;
public class PhoneBook {
private static final String FILE_NAME = "phonebook.txt";
private static final String[] ATTRIBUTES = {"Name", "Phone Number"};
private static final String DELIMITER = ",";
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Please select an option:");
System.out.println("1. Add Entry");
System.out.println("2. Remove Entry");
System.out.println("3. Sorted Search");
System.out.println("4. Update Entry");
System.out.println("5. Display Entries");
System.out.println("6. Exit");
int option = scanner.nextInt();
scanner.nextLine(); // consume the \n character
switch (option) {
case 1:
addEntry(scanner);
break;
case 2:
removeEntry(scanner);
break;
case 3:
sortedSearch(scanner);
break;
case 4:
updateEntry(scanner);
break;
case 5:
displayEntries();
break;
case 6:
System.exit(0);
break;
default:
System.out.println("Invalid option!");
}
}
}
private static void addEntry(Scanner scanner) {
System.out.println("Please enter the name:");
String name = scanner.nextLine();
System.out.println("Please enter the phone number:");
String phoneNumber = scanner.nextLine();
try (PrintWriter writer = new PrintWriter(new FileWriter(FILE_NAME, true))) {
writer.println(name + DELIMITER + phoneNumber);
System.out.println("Entry added successfully!");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
private static void removeEntry(Scanner scanner) {
System.out.println("Please enter the name or phone number to remove:");
String query = scanner.nextLine();
List<String> lines = readAllLines();
boolean found = false;
try (PrintWriter writer = new PrintWriter(new FileWriter(FILE_NAME))) {
for (String line : lines) {
String[] parts = line.split(DELIMITER);
if (!parts[0].equals(query) && !parts[1].equals(query)) {
writer.println(line);
} else {
found = true;
}
}
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
if (found) {
System.out.println("Entry removed successfully!");
} else {
System.out.println("Entry not found!");
}
}
private static void sortedSearch(Scanner scanner) {
System.out.println("Please select an attribute to sort by:");
for (int i = 0; i < ATTRIBUTES.length; i++) {
System.out.println((i + 1) + ". " + ATTRIBUTES[i]);
}
int attributeIndex = scanner.nextInt() - 1;
scanner.nextLine(); // consume the \n character
System.out.println("Please enter the search query:");
String query = scanner.nextLine();
List<String> lines = readAllLines();
List<String[]> entries = new ArrayList<>();
for (String line : lines) {
String[] parts = line.split(DELIMITER);
entries.add(parts);
}
Collections.sort(entries, new Comparator<String[]>() {
@Override
public int compare(String[] o1, String[] o2) {
return o1[attributeIndex].compareTo(o2[attributeIndex]);
}
});
boolean found = false;
for (String[] entry : entries) {
if (entry[attributeIndex].contains(query)) {
System.out.println(entry[0] + " " + entry[1]);
found = true;
}
}
if (!found) {
System.out.println("No matching entries found!");
}
}
private static void updateEntry(Scanner scanner) {
System.out.println("Please enter the name or phone number to update:");
String query = scanner.nextLine();
List<String> lines = readAllLines();
boolean found = false;
try (PrintWriter writer = new PrintWriter(new FileWriter(FILE_NAME))) {
for (String line : lines) {
String[] parts = line.split(DELIMITER);
if (!parts[0].equals(query) && !parts[1].equals(query)) {
writer.println(line);
} else {
System.out.println("Please enter the new name:");
String name = scanner.nextLine();
System.out.println("Please enter the new phone number:");
String phoneNumber = scanner.nextLine();
writer.println(name + DELIMITER + phoneNumber);
found = true;
}
}
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
if (found) {
System.out.println("Entry updated successfully!");
} else {
System.out.println("Entry not found!");
}
}
private static void displayEntries() {
List<String> lines = readAllLines();
for (String line : lines) {
String[] parts = line.split(DELIMITER);
System.out.println(parts[0] + " " + parts[1]);
}
}
private static List<String> readAllLines() {
List<String> lines = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(FILE_NAME))) {
String line;
while ((line = reader.readLine()) != null) {
lines.add(line);
}
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
return lines;
}
}
```
注意,这只是一个简单的示例代码,可能需要根据具体需求进行修改和优化。此外,文件格式和路径也需要根据实际情况进行设置。
相关推荐
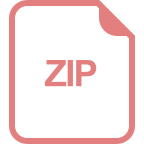
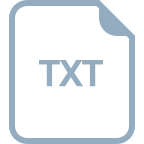
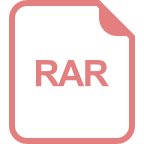











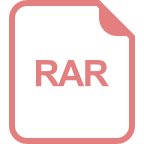
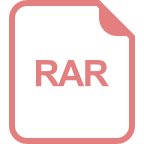
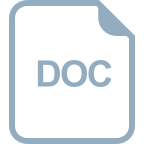