C# ftp是否存在相同文件
时间: 2023-10-19 14:59:40 浏览: 43
您好,要判断 FTP 服务器上是否存在相同的文件,可以使用 FTP 协议中的 SIZE 命令获取文件大小,如果文件大小相同,则判断为相同文件。以下是 C# 的示例代码:
```csharp
using System.Net;
// 获取文件大小
public static long GetFileSize(string url, string username, string password)
{
FtpWebRequest request = (FtpWebRequest)WebRequest.Create(url);
request.Method = WebRequestMethods.Ftp.GetFileSize;
request.Credentials = new NetworkCredential(username, password);
using (FtpWebResponse response = (FtpWebResponse)request.GetResponse())
{
return response.ContentLength;
}
}
// 判断文件是否存在
public static bool FileExists(string url, long size, string username, string password)
{
FtpWebRequest request = (FtpWebRequest)WebRequest.Create(url);
request.Method = WebRequestMethods.Ftp.GetFileSize;
request.Credentials = new NetworkCredential(username, password);
try
{
using (FtpWebResponse response = (FtpWebResponse)request.GetResponse())
{
long fileSize = response.ContentLength;
return fileSize == size;
}
}
catch (WebException ex)
{
FtpWebResponse response = (FtpWebResponse)ex.Response;
if (response.StatusCode == FtpStatusCode.ActionNotTakenFileUnavailable)
{
return false;
}
else
{
throw;
}
}
}
// 示例用法
string url = "ftp://ftp.example.com/path/to/file.txt";
string username = "username";
string password = "password";
long size = GetFileSize(url, username, password);
bool exists = FileExists(url, size, username, password);
if (exists)
{
Console.WriteLine("File exists.");
}
else
{
Console.WriteLine("File does not exist.");
}
```
请注意,上述代码仅供参考,具体实现可能因 FTP 服务器的不同而有所差异。
相关推荐
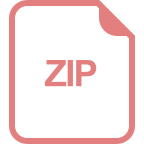
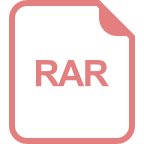














