4. 用继承的方法创建一个类,初步实现多态操作。c++代码
时间: 2024-05-16 10:14:03 浏览: 19
下面是一个简单的 C++ 代码示例,用继承的方法创建了一个 Shape 类,它有两个派生类:Circle 和 Rectangle,实现了多态操作。
```c++
#include <iostream>
using namespace std;
// Shape 类
class Shape {
protected:
int width, height;
public:
Shape( int a = 0, int b = 0) {
width = a;
height = b;
}
virtual int area() {
cout << "Parent class area :" <<endl;
return 0;
}
};
// Circle 类
class Circle: public Shape {
public:
Circle( int a = 0, int b = 0) : Shape(a, b) { }
int area () {
cout << "Circle class area :" <<endl;
return (width * height * 3);
}
};
// Rectangle 类
class Rectangle: public Shape{
public:
Rectangle( int a = 0, int b = 0) : Shape(a, b) { }
int area () {
cout << "Rectangle class area :" <<endl;
return (width * height);
}
};
int main() {
Shape *shape;
Circle circle(10,7);
Rectangle rectangle(5,6);
// 存储 Circle 的地址
shape = &circle;
// 调用 Circle 的 area
shape->area();
// 存储 Rectangle 的地址
shape = &rectangle;
// 调用 Rectangle 的 area
shape->area();
return 0;
}
```
输出结果为:
```
Circle class area :
Rectangle class area :
```
在这个例子中,Shape 类是一个基类,Circle 和 Rectangle 类是它的派生类。通过在 Shape 类中定义一个虚函数 area(),在派生类中分别重写该函数,实现了多态操作。在主函数中,分别创建了 Circle 和 Rectangle 的对象,然后用一个指向 Shape 类的指针分别指向这两个对象,调用 area() 函数,输出了不同的结果。
相关推荐
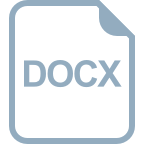
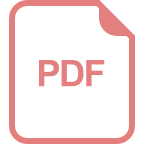
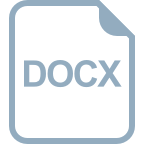
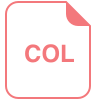













