创建一个名为Books的数据库,并在其中建立一个名为Book的表,字段包括书名、作者、出版社、出版时间和ISBN。编写一个应用程序,运用JDBC在该数据库中实现增加、删除、修改数据、查询数据的功能
时间: 2024-05-13 11:19:45 浏览: 48
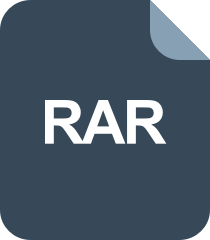
JDB.jar包(可进行JDBC的连接,对表中数据执行增,删,改,查操作)

首先,需要安装JDBC驱动程序,然后创建一个Java应用程序来连接到数据库,并执行增加、删除、修改和查询数据的操作。
以下是一个简单的示例代码:
```java
import java.sql.*;
public class BooksApp {
// JDBC连接URL
static final String DB_URL = "jdbc:mysql://localhost/Books";
// 数据库用户名和密码
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
// 注册JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 打开一个连接
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
// 执行增加数据操作
System.out.println("Inserting records into the table...");
stmt = conn.createStatement();
String sql = "INSERT INTO Book (title, author, publisher, publish_date, isbn) " +
"VALUES ('The Great Gatsby', 'F. Scott Fitzgerald', 'Charles Scribner\'s Sons', '1925-04-10', '978-0-684-80146-2')";
stmt.executeUpdate(sql);
// 执行修改数据操作
System.out.println("Updating records in the table...");
sql = "UPDATE Book SET author='Jane Austen' WHERE title='Pride and Prejudice'";
stmt.executeUpdate(sql);
// 执行删除数据操作
System.out.println("Deleting records from the table...");
sql = "DELETE FROM Book WHERE title='The Catcher in the Rye'";
stmt.executeUpdate(sql);
// 执行查询数据操作
System.out.println("Reading records from the table...");
sql = "SELECT title, author, publisher, publish_date, isbn FROM Book";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
// 从结果集中获取数据
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
String publishDate = rs.getString("publish_date");
String isbn = rs.getString("isbn");
// 打印数据
System.out.print("Title: " + title);
System.out.print(", Author: " + author);
System.out.print(", Publisher: " + publisher);
System.out.print(", Publish Date: " + publishDate);
System.out.println(", ISBN: " + isbn);
}
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
// 处理JDBC错误
se.printStackTrace();
}catch(Exception e){
// 处理Class.forName错误
e.printStackTrace();
}finally{
// 关闭资源
try{
if(stmt!=null) stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null) conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
以上代码演示了如何连接到名为Books的数据库,并在其中创建一个名为Book的表。然后,我们执行了增加、修改、删除和查询数据的操作。
注:以上代码中的用户名和密码是需要替换为实际的用户名和密码。
阅读全文
相关推荐
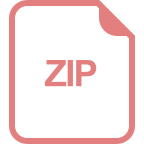








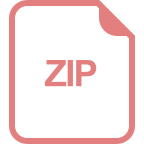
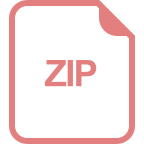
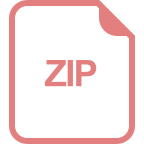