C语言链表实现停车场管理系统代码
时间: 2023-09-01 18:12:56 浏览: 112
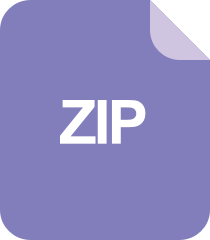
C语言停车场管理系统(完整代码)

以下是一个简单的停车场管理系统代码,使用了单向链表实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 20
typedef struct car {
char license[MAX_LENGTH];
struct car *next;
} Car;
Car *parking_lot = NULL;
void init_parking_lot() {
parking_lot = NULL;
}
int is_parking_lot_empty() {
return parking_lot == NULL;
}
void add_car_to_parking_lot(char *license) {
Car *new_car = (Car *) malloc(sizeof(Car));
strncpy(new_car->license, license, MAX_LENGTH);
new_car->next = NULL;
if (is_parking_lot_empty()) {
parking_lot = new_car;
} else {
Car *last_car = parking_lot;
while (last_car->next != NULL) {
last_car = last_car->next;
}
last_car->next = new_car;
}
printf("%s has been added to the parking lot.\n", license);
}
void remove_car_from_parking_lot(char *license) {
if (is_parking_lot_empty()) {
printf("Parking lot is empty.\n");
} else {
Car *current_car = parking_lot;
Car *previous_car = NULL;
while (current_car != NULL && strcmp(current_car->license, license) != 0) {
previous_car = current_car;
current_car = current_car->next;
}
if (current_car != NULL) {
if (previous_car != NULL) {
previous_car->next = current_car->next;
} else {
parking_lot = current_car->next;
}
free(current_car);
printf("%s has been removed from the parking lot.\n", license);
} else {
printf("%s is not in the parking lot.\n", license);
}
}
}
void print_parking_lot() {
if (is_parking_lot_empty()) {
printf("Parking lot is empty.\n");
} else {
printf("Cars in the parking lot:\n");
Car *current_car = parking_lot;
while (current_car != NULL) {
printf("- %s\n", current_car->license);
current_car = current_car->next;
}
}
}
int main() {
init_parking_lot();
add_car_to_parking_lot("ABC123");
add_car_to_parking_lot("DEF456");
print_parking_lot();
remove_car_from_parking_lot("ABC123");
print_parking_lot();
remove_car_from_parking_lot("GHI789");
return 0;
}
```
在上面的代码中,我们使用 `Car` 结构体来表示停车场中的汽车,其中包括车牌号 `license` 和指向下一辆车的指针 `next`。`parking_lot` 是一个指向第一辆车的指针,当停车场为空时,它指向 `NULL`。
在 `add_car_to_parking_lot` 函数中,我们创建一个新的 `Car` 结构体,并将车牌号复制到 `license` 字段中。然后,我们将新的汽车添加到停车场的末尾。如果停车场为空,我们将 `parking_lot` 指向新的汽车;否则,我们找到最后一辆汽车,并将其 `next` 指向新的汽车。
在 `remove_car_from_parking_lot` 函数中,我们首先检查停车场是否为空。如果停车场为空,我们输出一条消息。否则,我们遍历链表,查找车牌号与参数 `license` 相同的汽车。如果找到了这辆车,我们将它从链表中删除。如果找不到这辆车,我们也输出一条消息。
最后,我们使用 `print_parking_lot` 函数打印停车场中的所有汽车。如果停车场为空,我们输出一条消息。否则,我们遍历链表,并输出每辆汽车的车牌号。
这只是一个简单的停车场管理系统代码,有很多可以改进的地方。例如,我们可以添加计费和付款功能,以及限制停车场的容量。
阅读全文
相关推荐
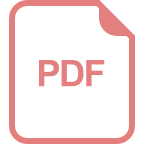
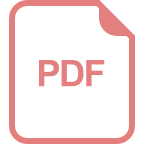













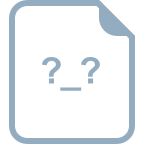
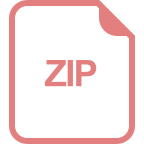
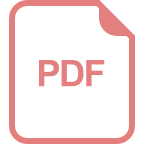