C语言数据结构的停车场管理系统代码
时间: 2023-09-01 18:07:44 浏览: 44
以下是一个简单的停车场管理系统的 C 语言代码,使用链表数据结构实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CAR_NUM 50 // 最大车位数
typedef struct Car {
char id[20]; // 车牌号
struct Car *next;
} Car;
Car *head = NULL; // 链表头指针
int car_num = 0; // 当前停车场车辆数
// 添加车辆
void add_car()
{
if (car_num >= MAX_CAR_NUM) {
printf("停车场已满,无法停车!\n");
return;
}
Car *new_car = (Car *)malloc(sizeof(Car));
printf("请输入车牌号:");
scanf("%s", new_car->id);
new_car->next = head;
head = new_car;
car_num++;
printf("车辆 %s 停入停车场!\n", new_car->id);
}
// 删除车辆
void del_car()
{
if (car_num == 0) {
printf("停车场已空,无车辆可出!\n");
return;
}
char id[20];
printf("请输入出场车辆的车牌号:");
scanf("%s", id);
Car *p = head;
Car *pre = NULL;
while (p != NULL && strcmp(p->id, id) != 0) {
pre = p;
p = p->next;
}
if (p == NULL) {
printf("未找到车牌号为 %s 的车辆!\n", id);
return;
}
if (pre == NULL) {
head = p->next;
} else {
pre->next = p->next;
}
free(p);
car_num--;
printf("车辆 %s 已成功出场!\n", id);
}
// 查询车辆
void query_car()
{
if (car_num == 0) {
printf("停车场已空,无车辆可查询!\n");
return;
}
char id[20];
printf("请输入要查询的车牌号:");
scanf("%s", id);
Car *p = head;
while (p != NULL && strcmp(p->id, id) != 0) {
p = p->next;
}
if (p == NULL) {
printf("未找到车牌号为 %s 的车辆!\n", id);
} else {
printf("车牌号为 %s 的车辆在停车场中!\n", id);
}
}
// 显示停车场信息
void show_info()
{
printf("当前停车场车辆数:%d\n", car_num);
printf("停车场车辆列表:\n");
Car *p = head;
while (p != NULL) {
printf("%s\n", p->id);
p = p->next;
}
}
int main()
{
int choice;
while (1) {
printf("\n********** 停车场管理系统 **********\n");
printf("1.添加车辆 2.删除车辆 3.查询车辆 4.显示信息 0.退出\n");
printf("请输入数字选择功能:");
scanf("%d", &choice);
switch (choice) {
case 0:
printf("欢迎下次使用!\n");
return 0;
case 1:
add_car();
break;
case 2:
del_car();
break;
case 3:
query_car();
break;
case 4:
show_info();
break;
default:
printf("输入错误,请重新输入!\n");
break;
}
}
return 0;
}
```
这个程序使用链表实现停车场的管理,每个车辆节点包含车牌号和指向下一个车辆节点的指针。程序提供添加、删除、查询和显示信息等功能。用户可以通过输入数字选择需要执行的功能。程序中使用了 malloc() 和 free() 函数动态分配和释放内存,可以有效地管理车辆信息。
相关推荐
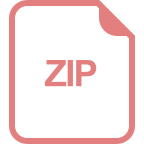












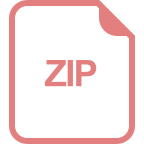