创建一个有6个结点的单向链表,实现增加、删除、查找、移动、显示结点的基本功能 用c语言
时间: 2024-01-27 08:06:30 浏览: 32
以下是一个简单的用C语言实现单向链表的示例,包含了增加、删除、查找、移动和显示结点的基本功能。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
struct Node {
int data;
struct Node* next;
};
// 创建新结点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表尾部添加新结点
void addNode(struct Node** headRef, int data) {
struct Node* newNode = createNode(data);
if (*headRef == NULL) {
*headRef = newNode;
return;
}
struct Node* lastNode = *headRef;
while (lastNode->next != NULL) {
lastNode = lastNode->next;
}
lastNode->next = newNode;
}
// 在链表中删除指定值的结点
void deleteNode(struct Node** headRef, int data) {
struct Node* temp = *headRef;
struct Node* prev = NULL;
if (temp != NULL && temp->data == data) {
*headRef = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != data) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
return;
}
prev->next = temp->next;
free(temp);
}
// 查找指定值的结点
struct Node* searchNode(struct Node* head, int data) {
while (head != NULL) {
if (head->data == data) {
return head;
}
head = head->next;
}
return NULL;
}
// 移动指定位置的结点到链表头部
void moveToHead(struct Node** headRef, int position) {
if (position <= 1) {
return;
}
struct Node* temp = *headRef;
struct Node* prev = NULL;
int i = 1;
while (temp != NULL && i < position) {
prev = temp;
temp = temp->next;
i++;
}
if (temp == NULL) {
return;
}
prev->next = temp->next;
temp->next = *headRef;
*headRef = temp;
}
// 显示链表中所有结点的值
void displayList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
// 添加结点
addNode(&head, 1);
addNode(&head, 2);
addNode(&head, 3);
addNode(&head, 4);
addNode(&head, 5);
addNode(&head, 6);
// 显示结点
printf("Initial list: ");
displayList(head);
// 删除结点
deleteNode(&head, 3);
printf("After deleting node with value 3: ");
displayList(head);
// 查找结点
struct Node* foundNode = searchNode(head, 5);
if (foundNode != NULL) {
printf("Node with value 5 found!\n");
} else {
printf("Node with value 5 not found!\n");
}
// 移动结点
moveToHead(&head, 4);
printf("After moving node at position 4 to the head: ");
displayList(head);
return 0;
}
```
输出结果如下:
```
Initial list: 1 2 3 4 5 6
After deleting node with value 3: 1 2 4 5 6
Node with value 5 found!
After moving node at position 4 to the head: 5 1 2 4 6
```
相关推荐
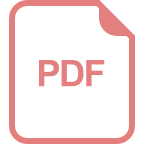
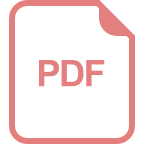














