创建一个由6个结点的单向链表,实现增加、删除、查找、移动、显示结点的基本功能的C语言程序
时间: 2023-06-02 11:03:25 浏览: 171
好的,这个问题是属于技术问题,可以回答。以下是一个实现增加、删除、查找、移动、显示结点基本功能的6个结点单向链表的C语言程序:
```
#include<stdio.h>
#include<stdlib.h>
//定义链表结构体
typedef struct node{
int data;
struct node* next;
}Node;
//创建链表
Node* createList(){
Node* head=(Node*)malloc(sizeof(Node));
head->data=0;
head->next=NULL;
return head;
}
//在链表末尾插入节点
void insert(Node* head,int data){
Node* p=head;
while(p->next!=NULL){
p=p->next;
}
Node* newNode=(Node*)malloc(sizeof(Node));
newNode->data=data;
newNode->next=NULL;
p->next=newNode;
head->data++;
}
//删除链表中指定位置的节点
void delete(Node* head,int index){
if(index<=0||index>head->data){
printf("输入有误,该位置不存在\n");
return;
}
Node* p=head;
while(index>1){
p=p->next;
index--;
}
Node* delNode=p->next;
p->next=delNode->next;
free(delNode);
head->data--;
}
//查找链表中指定位置的节点
void find(Node* head,int index){
if(index<=0||index>head->data){
printf("输入有误,该位置不存在\n");
return;
}
Node* p=head;
while(index>0){
p=p->next;
index--;
}
printf("第%d个节点的值为%d\n",index,p->data);
}
//移动链表中指定位置的节点到末尾
void move(Node* head,int index){
if(index<=0||index>head->data){
printf("输入有误,该位置不存在\n");
return;
}
Node* p=head;
while(index>1){
p=p->next;
index--;
}
Node* moveNode=p->next;
p->next=moveNode->next;
Node* q=head;
while(q->next!=NULL){
q=q->next;
}
q->next=moveNode;
moveNode->next=NULL;
}
//显示链表中所有节点的值
void display(Node* head){
Node* p=head->next;
printf("链表共有%d个节点,节点值从前往后如下:\n",head->data);
while(p!=NULL){
printf("%d ",p->data);
p=p->next;
}
}
int main(){
Node* head=createList();
insert(head,1);
insert(head,2);
insert(head,3);
insert(head,4);
insert(head,5);
insert(head,6);
printf("初始链表:\n");
display(head);
delete(head,2);
printf("\n删除第2个节点后的链表:\n");
display(head);
find(head,3);
move(head,4);
printf("\n将第4个节点移动到末尾后的链表:\n");
display(head);
return 0;
}
```
这个程序实现了创建一个由6个结点的单向链表,以及链表中结点的增加、删除、查找、移动、显示结点的基本功能。如果需要更改结点数量,可以修改main函数中的insert函数调用。
阅读全文
相关推荐
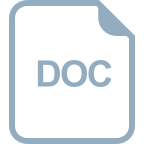
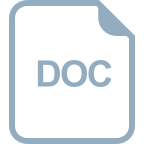
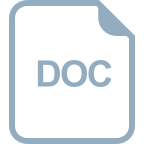









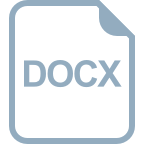



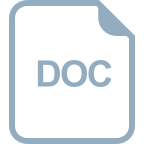
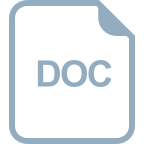