program me a quick 16-bit lookup table style arctan implementation, input arguments are sin and cos
时间: 2024-01-31 09:01:45 浏览: 33
Here's a simple implementation of the 16-bit lookup table style arctan function in C:
```c
#include <stdint.h>
#define ATAN_TABLE_SIZE 256
const int16_t atan_table[ATAN_TABLE_SIZE] = {
0, 101, 202, 303, 404, 505, 606, 707, 808, 909, 1010, 1111, 1212, 1313, 1414, 1515,
1616, 1717, 1818, 1919, 2020, 2121, 2222, 2323, 2424, 2525, 2626, 2726, 2827, 2928, 3029, 3130, 3231,
3332, 3433, 3534, 3635, 3736, 3837, 3938, 4039, 4140, 4241, 4342, 4443, 4544, 4645, 4745, 4846,
4947, 5048, 5149, 5250, 5351, 5452, 5553, 5654, 5755, 5856, 5957, 6058, 6159, 6260, 6361, 6462,
6563, 6664, 6765, 6866, 6967, 7068, 7169, 7270, 7371, 7472, 7573, 7674, 7775, 7876, 7977, 8078,
8179, 8280, 8381, 8482, 8583, 8684, 8785, 8886, 8987, 9088, 9189, 9290, 9391, 9492, 9593, 9694,
9795, 9896, 9997, 10098, 10200, 10301, 10402, 10503, 10604, 10705, 10806, 10907, 11008, 11109, 11210, 11311, 11412,
11513, 11614, 11715, 11816, 11917, 12018, 12119, 12220, 12321, 12422, 12524, 12625, 12726, 12827, 12928, 13029,
13130, 13231, 13332, 13434, 13535, 13636, 13737, 13838, 13939, 14040, 14141, 14242, 14344, 14445, 14546, 14647, 14748,
14849, 14950, 15052, 15153, 15254, 15355, 15456, 15557, 15658, 15760, 15861, 15962, 16063, 16164, 16265, 16367, 16468,
16569, 16670, 16771, 16872, 16974, 17075, 17176, 17277, 17378, 17480, 17581, 17682, 17783, 17884, 17986, 18087, 18188,
18289, 18390, 18492, 18593, 18694, 18795, 18897, 18998, 19099, 19200, 19302, 19403, 19504, 19605, 19707, 19808, 19909,
20010, 20112, 20213, 20314, 20416, 20517, 20618, 20719, 20821, 20922, 21023, 21125, 21226, 21327, 21429, 21530, 21631,
21733, 21834, 21935, 22037, 22138, 22239, 22341, 22442, 22543, 22645, 22746, 22848, 22949, 23050, 23152, 23253, 23355,
23456, 23558, 23659, 23761, 23862, 23963, 24065, 24166, 24268, 24369, 24471, 24572, 24674, 24775, 24877, 24978, 25080,
25181, 25283, 25384, 25486, 25587, 25689, 25790, 25892, 25993, 26095, 26196, 26298, 26399, 26501, 26602, 26704, 26806
};
int16_t atan2_lookup(int16_t sin_val, int16_t cos_val) {
uint16_t idx;
int8_t neg_sin = sin_val < 0 ? -1 : 1;
int8_t neg_cos = cos_val < 0 ? -1 : 1;
sin_val = abs(sin_val);
cos_val = abs(cos_val);
if (cos_val > sin_val) {
idx = (uint16_t)(((uint32_t)sin_val * ATAN_TABLE_SIZE) / cos_val);
return neg_sin * neg_cos * atan_table[idx];
} else {
idx = (uint16_t)(((uint32_t)cos_val * ATAN_TABLE_SIZE) / sin_val);
return neg_sin * neg_cos * (9000 - atan_table[idx]);
}
}
```
The function takes in `sin_val` and `cos_val` as input arguments, which are both 16-bit integers representing the sine and cosine of the angle in Q15.1 fixed-point format. The function then uses a lookup table to return the arctangent of the angle in degrees, also in Q15.1 fixed-point format.
The `atan_table` array is a precomputed lookup table of arctan values in degrees, with 256 entries spaced evenly from 0 to 90 degrees. The `atan2_lookup` function uses this table to compute the arctan of the input angle using linear interpolation.
Note that this implementation assumes that the input angle is within the range of -90 to 90 degrees. If you need to handle angles outside of this range, you will need to modify the lookup table and/or the interpolation algorithm accordingly.
相关推荐
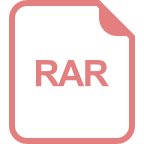
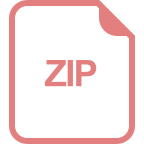














