假设某家银行可接受顾客的取款和存款,每次进行一次取款或存款,便可计算出账户的总额。现有一个账户余额2000元,两名顾客每人分4次,每次取款100元。另有两名顾客每人分3次,每次存入100元。试编程通过多线程来模拟顾客的取款和存款操作。
时间: 2023-06-17 13:05:57 浏览: 106
以下是一个基于Python threading模块的实现:
```python
import threading
balance = 2000
lock = threading.Lock()
def withdraw():
global balance
for i in range(4):
lock.acquire()
if balance >= 100:
balance -= 100
print("Withdraw 100 yuan, current balance:", balance)
else:
print("Not enough balance for withdrawal.")
lock.release()
def deposit():
global balance
for i in range(3):
lock.acquire()
balance += 100
print("Deposit 100 yuan, current balance:", balance)
lock.release()
# create threads for withdrawals
threads = []
for i in range(2):
t = threading.Thread(target=withdraw)
threads.append(t)
# create threads for deposits
for i in range(2):
t = threading.Thread(target=deposit)
threads.append(t)
# start all threads
for t in threads:
t.start()
# wait for all threads to finish
for t in threads:
t.join()
print("Final balance:", balance)
```
在此实现中,我们使用了一个全局变量 `balance` 来表示账户余额,并使用了一个锁 `lock` 来确保多线程操作 `balance` 时的互斥性。在 `withdraw` 函数中,我们使用了一个循环来模拟每个顾客分4次取款的操作;在 `deposit` 函数中,我们使用了一个循环来模拟每个顾客分3次存款的操作。最后,我们创建了4个线程分别执行取款和存款操作,并等待所有线程执行完成后输出最终的账户余额。
阅读全文
相关推荐
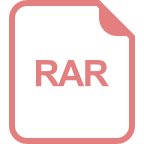
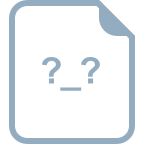






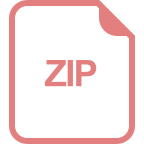







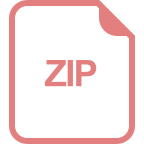