Java 假设某家银行可接受顾客的取款和存款,每次进行一次取款或存款,便可计算出账户的总额。现有一个账户余额2000元,两名顾客每人分4次,每次取款100元。另有两名顾客每人分3次,每次存入100元。试编程通过多线程来模拟顾客的取款和存款操作。
时间: 2024-03-19 17:45:38 浏览: 127
好的,以下是使用 Java 多线程来模拟顾客的取款和存款操作的代码:
```java
public class BankAccount {
private int balance = 2000;
public synchronized void deposit(int amount) {
balance += amount;
System.out.println("Deposited " + amount + ", current balance: " + balance);
}
public synchronized void withdraw(int amount) {
if (balance < amount) {
System.out.println("Not enough balance, current balance: " + balance);
return;
}
balance -= amount;
System.out.println("Withdrawn " + amount + ", current balance: " + balance);
}
}
public class Customer implements Runnable {
private BankAccount bankAccount;
private String name;
private int transactionType; // 1 for deposit, 2 for withdraw
private int transactionCount;
private int transactionAmount;
public Customer(BankAccount bankAccount, String name, int transactionType, int transactionCount, int transactionAmount) {
this.bankAccount = bankAccount;
this.name = name;
this.transactionType = transactionType;
this.transactionCount = transactionCount;
this.transactionAmount = transactionAmount;
}
@Override
public void run() {
for (int i = 0; i < transactionCount; i++) {
if (transactionType == 1) {
bankAccount.deposit(transactionAmount);
} else if (transactionType == 2) {
bankAccount.withdraw(transactionAmount);
}
try {
Thread.sleep(1000); // wait for 1 second before next transaction
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(name + " finished all transactions.");
}
}
public class Main {
public static void main(String[] args) {
BankAccount bankAccount = new BankAccount();
// create 4 customers who withdraw 100 yuan for 4 times
Thread t1 = new Thread(new Customer(bankAccount, "Customer 1", 2, 4, 100));
Thread t2 = new Thread(new Customer(bankAccount, "Customer 2", 2, 4, 100));
Thread t3 = new Thread(new Customer(bankAccount, "Customer 3", 2, 4, 100));
Thread t4 = new Thread(new Customer(bankAccount, "Customer 4", 2, 4, 100));
// create 2 customers who deposit 100 yuan for 3 times
Thread t5 = new Thread(new Customer(bankAccount, "Customer 5", 1, 3, 100));
Thread t6 = new Thread(new Customer(bankAccount, "Customer 6", 1, 3, 100));
// start all threads
t1.start();
t2.start();
t3.start();
t4.start();
t5.start();
t6.start();
}
}
```
代码的核心是 `BankAccount` 类和 `Customer` 类。`BankAccount` 类表示银行账户,有两个方法 `deposit` 和 `withdraw`,分别表示存款和取款。这两个方法都是同步方法,保证了多个线程同时操作账户时不会出现问题。
`Customer` 类表示顾客,有 5 个属性:`bankAccount` 表示顾客所属的银行账户,`name` 表示顾客的名字,`transactionType` 表示顾客的交易类型(1 表示存款,2 表示取款),`transactionCount` 表示顾客的交易次数,`transactionAmount` 表示每次交易的金额。`Customer` 类实现了 `Runnable` 接口,重写了 `run` 方法,表示顾客的操作。每个顾客在 `run` 方法中通过调用 `deposit` 或 `withdraw` 方法来进行交易。
在 `Main` 类中,我们创建了 6 个线程,分别表示 6 个顾客。其中前 4 个顾客每人分 4 次取款,后 2 个顾客每人分 3 次存款。最后,我们启动了这 6 个线程,让它们同时进行交易。
运行程序,可以看到多个顾客交替进行存款和取款操作,并且账户余额正确。
阅读全文
相关推荐
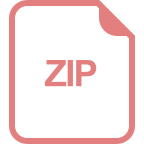
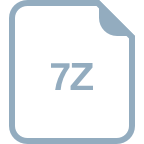














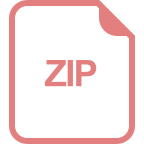
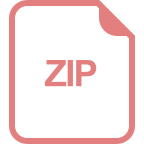