java外部存储设备写入认证
时间: 2023-05-19 21:04:26 浏览: 44
Java外部存储设备写入认证是指在Java程序中,对外部存储设备进行写入操作时需要进行认证,以确保数据的安全性和完整性。具体的认证方式可以根据实际需求进行选择,例如使用数字签名、加密算法等。如果需要更详细的信息,可以查阅相关的Java文档或者参考相关的技术资料。
相关问题
java外部存储设备写入认证,给个示例
可以使用以下代码示例来进行Java外部存储设备的写入认证:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class ExternalStorageAuthenticator {
private static final String AUTHENTICATION_KEY = "my_secret_key";
public static void main(String[] args) throws IOException, NoSuchAlgorithmException {
File externalStorage = new File("/mnt/sdcard/my_file.txt");
String dataToWrite = "Hello, world!";
byte[] authenticationHash = getAuthenticationHash(dataToWrite);
FileOutputStream outputStream = new FileOutputStream(externalStorage);
outputStream.write(dataToWrite.getBytes(StandardCharsets.UTF_8));
outputStream.write(authenticationHash);
outputStream.close();
}
private static byte[] getAuthenticationHash(String data) throws NoSuchAlgorithmException {
MessageDigest messageDigest = MessageDigest.getInstance("SHA-256");
messageDigest.update(data.getBytes(StandardCharsets.UTF_8));
messageDigest.update(AUTHENTICATION_KEY.getBytes(StandardCharsets.UTF_8));
return messageDigest.digest();
}
}
```
这个示例演示了如何将数据写入外部存储设备,并使用SHA-256哈希算法对数据进行认证。在这个示例中,我们使用了一个预定义的认证密钥来计算哈希值。在实际应用中,你应该使用一个更安全的密钥来保护你的数据。
java外部存储设备写入认证 给个完整的示例
以下是一个 Java 外部存储设备写入认证的示例代码:
```java
import java.io.*;
import java.security.*;
public class ExternalStorageAuthenticator {
private static final String AUTH_FILE = "/mnt/sdcard/auth.txt";
private static final String AUTH_ALGORITHM = "SHA-256";
private static final String AUTH_KEY = "my_secret_key";
public static boolean authenticate(String data) {
try {
MessageDigest md = MessageDigest.getInstance(AUTH_ALGORITHM);
md.update(AUTH_KEY.getBytes());
byte[] keyHash = md.digest();
md.reset();
md.update(data.getBytes());
byte[] dataHash = md.digest();
byte[] authHash = new byte[keyHash.length + dataHash.length];
System.arraycopy(keyHash, 0, authHash, 0, keyHash.length);
System.arraycopy(dataHash, 0, authHash, keyHash.length, dataHash.length);
byte[] storedHash = readAuthFile();
return MessageDigest.isEqual(authHash, storedHash);
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
private static byte[] readAuthFile() throws IOException {
File file = new File(AUTH_FILE);
if (!file.exists()) {
throw new FileNotFoundException("Auth file not found");
}
byte[] buffer = new byte[(int) file.length()];
FileInputStream fis = new FileInputStream(file);
fis.read(buffer);
fis.close();
return buffer;
}
}
```
这个示例代码演示了如何使用 SHA-256 算法对数据进行哈希,然后将哈希值与一个密钥的哈希值拼接起来,最后将拼接后的哈希值与存储在外部存储设备上的认证哈希值进行比较,以实现认证的功能。
阅读全文
相关推荐
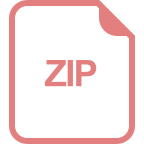
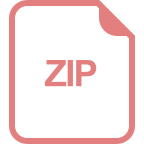
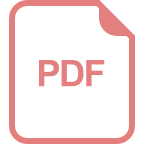
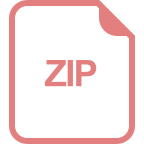
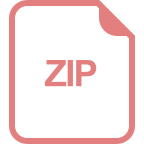
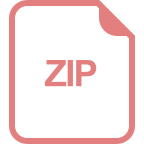
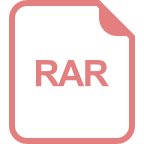
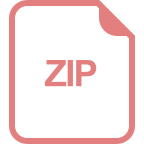
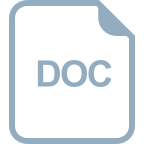
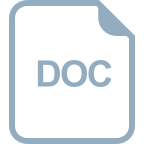



