使用java获取xml数据中的所有xpath
时间: 2024-03-09 10:49:52 浏览: 140
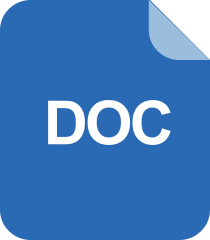
使用java对xml进行操作
使用Java获取XML数据中的所有XPath路径可以使用递归方法来实现,遍历XML节点并存储XPath路径。以下是一个示例代码:
```java
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathFactory;
import java.util.ArrayList;
import java.util.List;
public class XpathExtractor {
public static void main(String[] args) throws Exception {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("path/to/xml/file.xml");
List<String> xpaths = getXPaths(doc);
for (String xpath : xpaths) {
System.out.println(xpath);
}
}
public static List<String> getXPaths(Node node) throws Exception {
List<String> xpaths = new ArrayList<>();
XPathFactory xpathFactory = XPathFactory.newInstance();
XPath xpath = xpathFactory.newXPath();
// 获取节点的XPath
XPathExpression xpathExp = xpath.compile(getNodeXPath(node));
String xpathValue = (String) xpathExp.evaluate(node, XPathConstants.STRING);
xpaths.add(xpathValue);
// 遍历子节点
NodeList children = node.getChildNodes();
for (int i = 0; i < children.getLength(); i++) {
Node child = children.item(i);
if (child.getNodeType() == Node.ELEMENT_NODE) {
xpaths.addAll(getXPaths(child));
}
}
return xpaths;
}
private static String getNodeXPath(Node node) {
StringBuilder builder = new StringBuilder();
for (; node != null; node = node.getParentNode()) {
int index = getNodeIndex(node);
String nodeName = node.getNodeName();
builder.insert(0, "/" + nodeName + "[" + index + "]");
}
return builder.toString();
}
private static int getNodeIndex(Node node) {
int index = 1;
for (Node sibling = node.getPreviousSibling(); sibling != null; sibling = sibling.getPreviousSibling()) {
if (sibling.getNodeType() == node.getNodeType() && sibling.getNodeName().equals(node.getNodeName())) {
index++;
}
}
return index;
}
}
```
该示例代码使用了递归方法来获取XML数据中的所有XPath路径。getXPaths()方法接收一个XML节点,将该节点的XPath路径添加到一个列表中,并递归遍历节点的子节点,直到所有节点都被遍历完为止。该示例代码中还包括了getNodeXPath()和getNodeIndex()方法,用于获取节点的XPath路径和节点的索引。
阅读全文
相关推荐
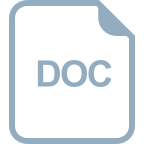
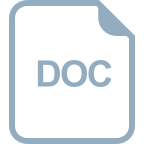
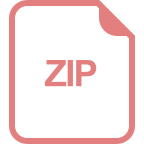
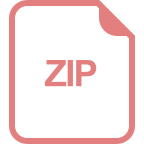
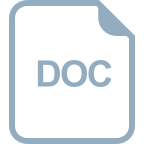
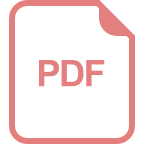
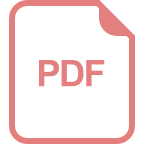
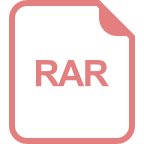
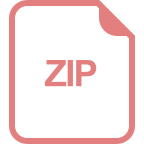
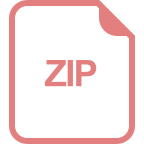
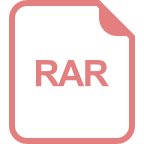
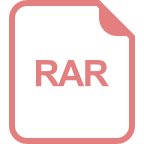
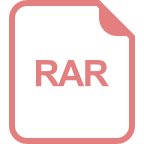
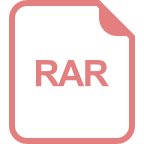
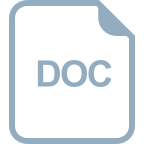
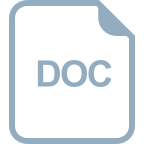

