设置有不同颜色的3d粒子球旋转
时间: 2023-09-26 15:07:28 浏览: 115
要设置不同颜色的3D粒子球旋转,可以在创建粒子模型时为每个粒子指定颜色。可以使用THREE.BufferAttribute或THREE.BufferGeometry等来设置颜色属性。
下面是一个示例代码,可以参考一下:
```html
<canvas id="canvas"></canvas>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r121/three.min.js"></script>
<script>
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const width = canvas.width = window.innerWidth;
const height = canvas.height = window.innerHeight;
// 创建场景、相机和渲染器
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, width / height, 0.1, 1000);
camera.position.z = 500;
const renderer = new THREE.WebGLRenderer({ canvas });
renderer.setSize(width, height);
// 创建粒子模型
const particleCount = 10000;
const particles = new THREE.BufferGeometry();
const positions = new Float32Array(particleCount * 3);
const colors = new Float32Array(particleCount * 3);
for (let i = 0; i < particleCount; i++) {
const x = Math.random() * 200 - 100;
const y = Math.random() * 200 - 100;
const z = Math.random() * 200 - 100;
positions[i * 3] = x;
positions[i * 3 + 1] = y;
positions[i * 3 + 2] = z;
colors[i * 3] = Math.random();
colors[i * 3 + 1] = Math.random();
colors[i * 3 + 2] = Math.random();
}
particles.setAttribute('position', new THREE.BufferAttribute(positions, 3));
particles.setAttribute('color', new THREE.BufferAttribute(colors, 3));
particles.computeBoundingSphere();
const particleMaterial = new THREE.PointsMaterial({
size: 3,
vertexColors: true,
});
const particleSystem = new THREE.Points(particles, particleMaterial);
scene.add(particleSystem);
// 实现粒子的旋转动画
function animate() {
requestAnimationFrame(animate);
particleSystem.rotation.x += 0.01;
particleSystem.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
</script>
```
这段代码创建了10000个随机位置和颜色的粒子模型,并使用THREE.BufferAttribute来设置颜色属性。最后使用THREE.PointsMaterial的vertexColors属性来开启顶点颜色功能,并将粒子模型添加到场景中。
阅读全文
相关推荐
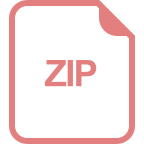
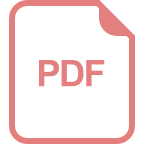
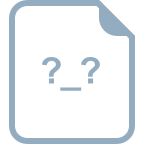

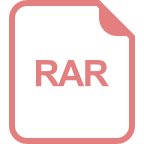
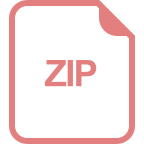
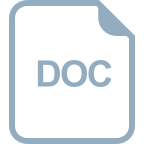
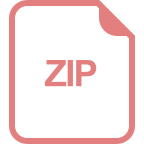
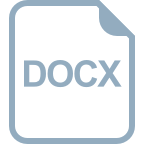
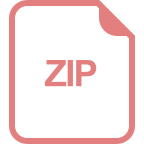
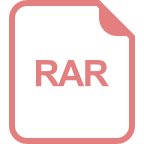
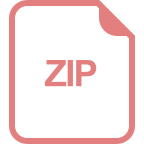
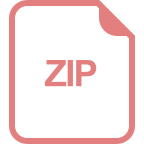
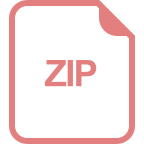
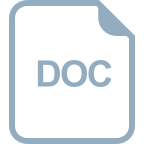
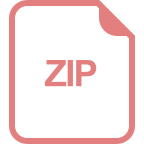
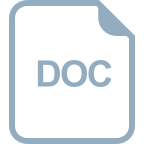
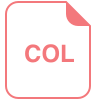