编程输入年份和月份,输出这一年的这个月份有多少天。c语言
时间: 2024-05-05 08:17:15 浏览: 147
实现如下:
```
#include <stdio.h>
int main() {
int year, month, days;
printf("请输入年份和月份(用空格隔开):");
scanf("%d %d", &year, &month);
if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days = 29;
} else {
days = 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
days = 30;
} else {
days = 31;
}
printf("%d年%d月有%d天。\n", year, month, days);
return 0;
}
```
首先,我们使用 `scanf()` 函数从用户输入中读取年份和月份。然后,使用条件语句来判断这个月有多少天。对于二月份,需要判断是否为闰年,如果是,则有 29 天,否则有 28 天。对于其它月份,根据月份的奇偶性来判断天数,其中 4、6、9、11 月份有 30 天,其余月份有 31 天。最后,使用 `printf()` 函数输出结果。
相关问题
请用c语言编程输入年份和月份,输出这一年的这个月份有多少天
在C语言中,你可以通过判断给定的年份和月份是否是闰年,并结合每个月的天数规则来计算特定月份的天数。以下是一个简单的示例程序:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 判断是否是闰年,闰年的条件:能被4整除但不能被100整除,或者是能被400整除
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
static const int month_days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 标准的非闰年月份数量
// 如果是闰年,二月有29天
if (is_leap_year(year)) {
month_days[1] = 29;
}
return month_days[month - 1];
}
int main() {
int year, month;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
// 检查输入的月份是否合法
if(month >= 1 && month <= 12) {
int days;
days = days_in_month(month, year);
printf("该年的%2d月共有%d天。\n", month, days);
} else {
printf("错误:月份必须在1到12之间。\n");
}
return 0;
}
```
在这个程序中,`days_in_month`函数根据输入的年份和月份确定当月的天数。注意我们使用了一个静态数组存储每个标准月份的天数,闰年情况会修改二月的天数。
c语言编程输入年份和月份,输出这一年的这个月份有多少天。 输入样例:
```c
#include <stdio.h>
int main() {
int year, month, days;
printf("请输入年份和月份:");
scanf("%d%d", &year, &month);
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days = 29;
} else {
days = 28;
}
break;
default:
printf("输入的月份有误!");
return 0;
}
printf("%d年%d月有%d天", year, month, days);
return 0;
}
```
阅读全文
相关推荐
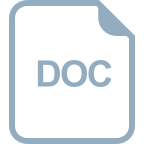
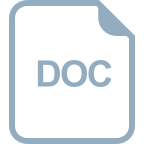
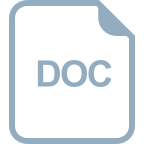





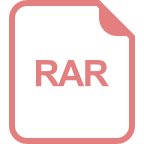







