假定某校有N各学生,系统可以根据学生每次上机时记录学号和上机时间,下机时自动计算费用。要求: (1)系统以菜单方式工作 (2)系统可以设置上机每小时的价格,默认为每小时1元, (3)系统可以输入功能N名学生的学号、专业,年级、姓名。 (4)计算功能:计算每个下机学生的上机费用,每小时1元。 (上机费用=上机时间* 价格 ,不足半小时按1小时的价格减半计算,超过半小时但不足一小时的按1小时计算) (5)查询功能:按条件(班级、学号、姓名)显示学生的上机时间。 (6)机器使用情况的显示(显示方式不限但要一目了然)用c语言简单表达
时间: 2023-12-23 12:02:07 浏览: 73
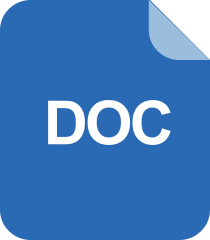
学生宿舍管理系统可行性研究报告

好的,我会尽力回答您的问题。根据您所描述的要求,这是一个基于学生上机记录的计费系统,具有以下功能:
1. 设置上机每小时的价格,默认为每小时1元;
2. 输入学生信息,包括学号、专业、年级、姓名;
3. 计算每个下机学生的上机费用,按照规定的计费方式进行计算;
4. 查询学生的上机时间,可以按照班级、学号、姓名等条件进行查询;
5. 显示机器使用情况,以便管理员实时掌握机器的使用情况。
以下是该系统的简单实现,基于C语言实现:
```
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX_STUDENT_NUM 1000
#define MAX_NAME_LEN 20
#define MAX_CLASS_LEN 20
struct Student {
char name[MAX_NAME_LEN];
char class[MAX_CLASS_LEN];
char major[MAX_NAME_LEN];
int grade;
int id;
int start_time;
int end_time;
float price;
};
struct Student students[MAX_STUDENT_NUM];
int num_students = 0;
float default_price = 1.0;
void add_student() {
if (num_students == MAX_STUDENT_NUM) {
printf("Error: Maximum number of students reached.\n");
return;
}
struct Student student;
printf("Please enter student information:\n");
printf("Name: ");
scanf("%s", student.name);
printf("Class: ");
scanf("%s", student.class);
printf("Major: ");
scanf("%s", student.major);
printf("Grade: ");
scanf("%d", &student.grade);
printf("ID: ");
scanf("%d", &student.id);
students[num_students++] = student;
}
void set_price() {
printf("Please enter the new price (default is %.2f): ", default_price);
scanf("%f", &default_price);
}
float calculate_price(float start_time, float end_time, float price_per_hour) {
float duration = end_time - start_time;
float price = duration * price_per_hour;
if (duration < 0.5) {
price = price_per_hour / 2.0;
} else if (duration < 1) {
price = price_per_hour;
} else {
price = ((int)duration) * price_per_hour + (duration - (int)duration) * price_per_hour;
}
return price;
}
void calculate_student_price(int index) {
struct Student student = students[index];
float price = calculate_price(student.start_time, student.end_time, default_price);
students[index].price = price;
}
void calculate_all_prices() {
for (int i = 0; i < num_students; i++) {
calculate_student_price(i);
}
}
void print_student(struct Student student) {
printf("%s, %s, %s, %d, %d, %.2f\n", student.name, student.class, student.major, student.grade, student.id, student.price);
}
void query_student() {
int choice;
printf("Please choose a query option:\n");
printf("1. Query by class\n");
printf("2. Query by ID\n");
printf("3. Query by name\n");
scanf("%d", &choice);
char query[MAX_NAME_LEN];
printf("Please enter the query string: ");
scanf("%s", query);
for (int i = 0; i < num_students; i++) {
struct Student student = students[i];
switch (choice) {
case 1:
if (strcmp(student.class, query) == 0) {
print_student(student);
}
break;
case 2:
if (student.id == atoi(query)) {
print_student(student);
}
break;
case 3:
if (strcmp(student.name, query) == 0) {
print_student(student);
}
break;
default:
printf("Error: Invalid query option.\n");
break;
}
}
}
void display_usage() {
printf("Usage:\n");
printf("1. Add student\n");
printf("2. Set price\n");
printf("3. Calculate prices\n");
printf("4. Query student\n");
printf("5. Display usage\n");
printf("6. Exit\n");
}
void display_machine_usage() {
printf("Machine usage:\n");
for (int i = 0; i < num_students; i++) {
struct Student student = students[i];
printf("%d: %s, %s, %d, %d, %.2f\n", i + 1, student.name, student.class, student.grade, student.id, student.price);
}
}
int main() {
int choice;
display_usage();
while (1) {
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_student();
break;
case 2:
set_price();
break;
case 3:
calculate_all_prices();
break;
case 4:
query_student();
break;
case 5:
display_usage();
break;
case 6:
printf("Exiting...\n");
return 0;
default:
printf("Error: Invalid choice.\n");
break;
}
display_machine_usage();
}
}
```
该程序中使用了一个简单的结构体来存储学生信息,包括姓名、班级、专业、年级、学号、上机开始时间、下机结束时间和上机费用。程序实现了添加学生、设置价格、计算费用、查询学生和显示机器使用情况等功能。注意,该程序仅供参考,可能需要根据实际需求进行修改和调整。
阅读全文
相关推荐
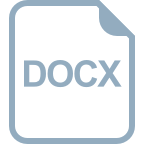
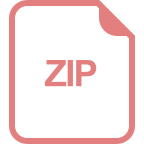














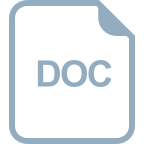
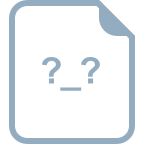