具体程序代码
时间: 2023-10-15 12:02:05 浏览: 145
以下是一个简单的Java事件处理ATM柜员机模拟程序的示例代码,您可以参考一下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ATM implements ActionListener {
private JFrame frame;
private JPanel panel;
private JLabel cardLabel, pinLabel;
private JTextField cardField, pinField;
private JButton enterButton;
private JButton withdrawButton, depositButton, balanceButton, exitButton;
private JLabel messageLabel;
private double balance = 0;
public ATM() {
// 创建窗口和面板
frame = new JFrame("ATM");
panel = new JPanel(new GridLayout(5, 2));
// 添加卡号和密码输入框
cardLabel = new JLabel("Card Number:");
panel.add(cardLabel);
cardField = new JTextField(20);
panel.add(cardField);
pinLabel = new JLabel("PIN:");
panel.add(pinLabel);
pinField = new JTextField(20);
panel.add(pinField);
// 添加登录按钮
enterButton = new JButton("Enter");
enterButton.addActionListener(this);
panel.add(enterButton);
// 添加操作按钮
withdrawButton = new JButton("Withdraw");
withdrawButton.addActionListener(this);
depositButton = new JButton("Deposit");
depositButton.addActionListener(this);
balanceButton = new JButton("Balance");
balanceButton.addActionListener(this);
exitButton = new JButton("Exit");
exitButton.addActionListener(this);
// 添加提示信息标签
messageLabel = new JLabel("");
// 将操作按钮和提示信息标签添加到面板中,但不显示
panel.add(withdrawButton);
panel.add(depositButton);
panel.add(balanceButton);
panel.add(exitButton);
panel.add(messageLabel);
withdrawButton.setVisible(false);
depositButton.setVisible(false);
balanceButton.setVisible(false);
exitButton.setVisible(false);
messageLabel.setVisible(false);
// 将面板添加到窗口中,并设置窗口大小
frame.getContentPane().add(panel);
frame.setSize(300, 200);
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String cardNumber = cardField.getText();
String pin = pinField.getText();
if (e.getSource() == enterButton) {
// 验证卡号和密码是否正确
if (cardNumber.equals("123456") && pin.equals("1234")) {
messageLabel.setText("Login successful!");
withdrawButton.setVisible(true);
depositButton.setVisible(true);
balanceButton.setVisible(true);
exitButton.setVisible(true);
messageLabel.setVisible(true);
} else {
messageLabel.setText("Invalid card number or PIN!");
messageLabel.setVisible(true);
}
} else if (e.getSource() == withdrawButton) {
// 执行取款操作
String amountString = JOptionPane.showInputDialog("Enter amount:");
if (amountString != null && !amountString.equals("")) {
double amount = Double.parseDouble(amountString);
if (amount > balance) {
messageLabel.setText("Insufficient balance!");
messageLabel.setVisible(true);
} else {
balance -= amount;
messageLabel.setText("Withdraw successfully! Balance: " + balance);
messageLabel.setVisible(true);
}
}
} else if (e.getSource() == depositButton) {
// 执行存款操作
String amountString = JOptionPane.showInputDialog("Enter amount:");
if (amountString != null && !amountString.equals("")) {
double amount = Double.parseDouble(amountString);
balance += amount;
messageLabel.setText("Deposit successfully! Balance: " + balance);
messageLabel.setVisible(true);
}
} else if (e.getSource() == balanceButton) {
// 执行查询余额操作
messageLabel.setText("Your balance is: " + balance);
messageLabel.setVisible(true);
} else if (e.getSource() == exitButton) {
// 执行退出操作
System.exit(0);
}
}
public static void main(String[] args) {
new ATM();
}
}
```
以上是一个简单的Java事件处理ATM柜员机模拟程序的示例代码,其中利用了Swing库的一些组件来实现界面的显示和事件的处理。程序运行后,会显示一个登录界面,用户输入卡号和密码后点击“Enter”按钮进行登录。登录成功后,会显示主菜单,包括取款、存款、查询余额和退出等选项。用户选择某个选项后,程序会触发相应的事件处理器,执行相应的操作。
阅读全文
相关推荐
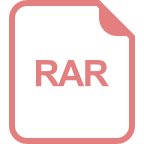
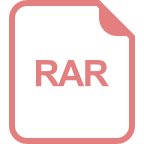
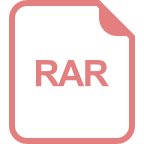
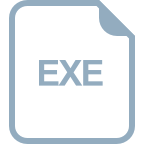
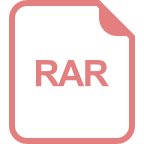
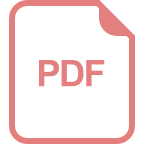
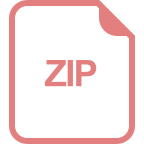
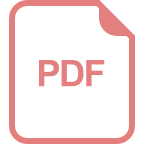
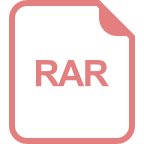
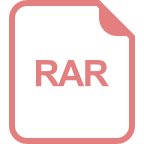
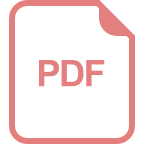