Python 通过四条线程并行控制Mq两个队列接受消息以及两个队列发送消息
时间: 2023-11-27 15:55:40 浏览: 115
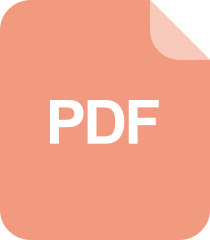
Python中线程的MQ消息队列实现以及消息队列的优点解析
下面是一个示例代码,使用 Python 的 threading 库来创建四个线程分别控制两个队列的接收和发送:
```python
import threading
import queue
# 创建两个队列,一个用于接收,一个用于发送
receive_queue = queue.Queue()
send_queue = queue.Queue()
# 定义线程函数,用于接收消息
def receive_message():
while True:
message = receive_queue.get() # 从队列中获取消息
# 处理消息的代码
print("Received message:", message)
# 定义线程函数,用于发送消息
def send_message():
while True:
message = input("Enter message to send: ")
send_queue.put(message) # 将消息放入队列中
# 处理消息的代码
print("Sent message:", message)
# 创建四个线程,分别控制接收和发送
receive_thread1 = threading.Thread(target=receive_message)
receive_thread2 = threading.Thread(target=receive_message)
send_thread1 = threading.Thread(target=send_message)
send_thread2 = threading.Thread(target=send_message)
# 启动四个线程
receive_thread1.start()
receive_thread2.start()
send_thread1.start()
send_thread2.start()
```
在上面的代码中,我们创建了两个队列,一个用于接收消息,一个用于发送消息。然后定义了两个线程函数,一个用于接收消息,一个用于发送消息。在接收消息的函数中,我们使用了一个无限循环来不断从队列中获取消息,并处理这些消息。在发送消息的函数中,我们使用了一个无限循环来等待用户输入消息,并将消息放入队列中。
最后,我们创建了四个线程,分别控制接收和发送。每个线程都调用对应的线程函数。启动四个线程后,就可以实现两个队列的并行控制了。
阅读全文
相关推荐
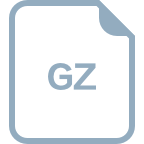
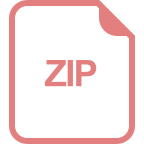















