用python和opencv写出二值化
时间: 2023-07-04 11:27:16 浏览: 73
好的,以下是用 Python 和 OpenCV 实现的二值化代码:
```python
import cv2
# 加载图像
img = cv2.imread("image.jpg")
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 应用阈值
threshold_value = 127
max_value = 255
_, binary = cv2.threshold(gray, threshold_value, max_value, cv2.THRESH_BINARY)
# 显示结果
cv2.imshow("Binary Image", binary)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们首先加载了一个图像,然后将其转换为灰度图像。接下来,我们应用了一个阈值,将图像二值化。最后,我们显示了二值化的结果。
在这个例子中,我们使用的阈值是 127,这意味着所有像素值小于 127 的像素都被设置为 0,所有像素值大于或等于 127 的像素都被设置为 255。如果你想要尝试不同的阈值,可以更改 `threshold_value` 变量的值。
相关问题
请写出python opencv 画出二值化图片百分之一的轮廓点具体代码
### 回答1:
import cv2
import numpy as np
# 读取图片
img = cv2.imread('binary_image.jpg')
# 灰度化
grayImage = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
ret,thresh = cv2.threshold(grayImage,127,255,cv2.THRESH_BINARY)
# 轮廓检测
image, contours, hierarchy = cv2.findContours(thresh,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE)
# 画出轮廓点,百分之一
sample_contour = np.vstack([contours[i] for i in range(0,len(contours),int(len(contours)/100))])
# 画图
cv2.drawContours(img, [sample_contour], 0, (0,255,0), 3)
# 显示
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
### 回答2:
以下是使用Python和OpenCV画出二值化图片百分之一的轮廓点的代码:
```python
import cv2
import numpy as np
# 读取二值化图像
image = cv2.imread("binary_image.png", 0)
# 检测轮廓
contours, _ = cv2.findContours(image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 计算百分之一的轮廓点数目
total_points = sum(len(contour) for contour in contours)
points_threshold = int(0.01 * total_points)
# 画出百分之一的轮廓点
for contour in contours:
# 如果轮廓点数小于阈值,则忽略该轮廓
if len(contour) < points_threshold:
continue
# 随机选择颜色
color = np.random.randint(0, 255, size=3).tolist()
# 画出轮廓点
for point in contour:
x, y = point[0]
cv2.circle(image, (x, y), 1, color, -1)
# 显示画出轮廓点的图像
cv2.imshow("Contour Points", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,在代码中的`"binary_image.png"`处需要替换为实际的二值化图像的文件路径。此代码会读取二值化图像,找到轮廓,计算出百分之一的轮廓点数目,然后随机选择颜色,在原始图像上画出百分之一的轮廓点,并显示结果图像。
### 回答3:
import cv2
# 读取图片
image = cv2.imread("image.jpg", 0)
# 进行二值化处理
_, binary = cv2.threshold(image, 127, 255, cv2.THRESH_BINARY)
# 查找轮廓
contours, _ = cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 计算轮廓点数量
contour_count = len(contours[0])
# 获取所需数量的轮廓点
sample_count = int(contour_count * 0.01)
sample_points = contours[0][:sample_count]
# 绘制轮廓点
for point in sample_points:
x, y = point[0]
cv2.circle(image, (x, y), 1, (255, 0, 0), -1)
# 显示结果
cv2.imshow("Contours", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
用python和opencv写出检测图片噪点并标识出来的代码
下面是一种用Python和OpenCV库检测图像噪点并标识出来的方法:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用高斯滤波去噪
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 使用二值化处理图像
_, thresh = cv2.threshold(blur, 127, 255, cv2.THRESH_BINARY)
# 找到噪点轮廓并画出矩形框
contours, _ = cv2.findContours(thresh, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
if w < 10 and h < 10:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 0, 255), 1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个脚本会打开名为“test.jpg”的图像文件,然后使用高斯滤波来减少噪点。接下来,它把图像转换成二值图像,使用findContours()函数来查找轮廓,并使用boundingRect()函数来查找矩形边界框。对于任何小于给定阈值的矩形,它会在图像上绘制红色矩形来标识它们。最后,它会显示图像并等待用户按下任意键来退出。
相关推荐
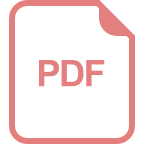
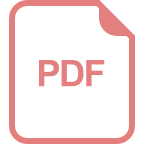











