C语言计算两个日期之间的天数差用vs
时间: 2023-10-14 19:15:24 浏览: 103
以下是使用C语言计算两个日期之间的天数差的示例代码:
```
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int daysInMonth(int year, int month) {
switch (month) {
case 2:
return (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
int daysBetweenDates(struct Date date1, struct Date date2) {
int days = 0;
if (date1.year == date2.year && date1.month == date2.month) {
days = date2.day - date1.day;
} else {
// Calculate days between date1 and the end of its month
days += daysInMonth(date1.year, date1.month) - date1.day;
// Calculate days between the start of date2's month and date2
days += date2.day;
// Calculate days between all the months in between
for (int year = date1.year; year < date2.year; year++) {
for (int month = date1.month + 1; month <= 12; month++) {
days += daysInMonth(year, month);
}
date1.month = 0; // reset month after first year
}
// Calculate days between all the months in the same year as date2
for (int month = 1; month < date2.month; month++) {
days += daysInMonth(date2.year, month);
}
}
return days;
}
int main() {
struct Date date1 = {2021, 9, 1};
struct Date date2 = {2021, 9, 30};
int days = daysBetweenDates(date1, date2);
printf("Days between %d/%d/%d and %d/%d/%d: %d\n", date1.year, date1.month, date1.day, date2.year, date2.month, date2.day, days);
return 0;
}
```
在这个示例中,我们定义了一个日期结构体,包含年份、月份和日份。我们还定义了一个函数,用于计算每个月的天数。然后,我们定义了一个计算日期之间天数差的函数,它使用了一个循环来计算两个日期之间所有的月份的天数,并对所有的月份进行累加。最后,我们在主函数中使用这个函数来计算两个日期之间的天数差,并打印输出结果。
请注意,这个示例代码仅适用于公元后的日期,不能用于公元前的日期计算。
阅读全文
相关推荐


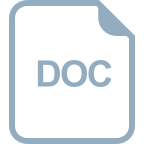





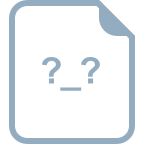
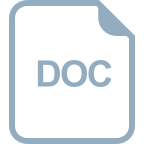




