python小游戏计时
时间: 2023-08-28 14:08:03 浏览: 181
可以使用Python内置的time模块来实现计时功能。具体实现方法如下:
1. 导入time模块:在代码的开头添加 `import time`
2. 获取当前时间:使用 `start_time = time.time()` 来获取当前时间,该函数返回一个浮点数,表示自1970年1月1日 00:00:00 UTC到现在的秒数。
3. 计算时间差:在游戏结束时,使用 `end_time = time.time()` 获取当前时间,然后计算时间差 `time_diff = end_time - start_time`,即为游戏所用时间。
下面是一个简单的计时器小游戏示例代码:
```python
import time
print("请按回车键开始计时,再次按回车键结束计时。")
input()
start_time = time.time()
print("计时开始!")
input("请按回车键停止计时。")
end_time = time.time()
time_diff = end_time - start_time
print("本次计时用时:{:.2f}秒".format(time_diff))
```
运行该程序后,按下回车键即可开始计时,再次按下回车键即可停止计时,程序会输出本次计时所用时间。
相关问题
python五子棋小游戏计时代码
在Python中创建一个简单的五子棋小游戏并添加计时功能,你可以使用`time`模块来记录游戏时间。以下是一个基本的框架,包括初始化棋盘、玩家轮流下棋、检查胜利条件以及计时:
```python
import random
import time
# 初始化棋盘
chess_board = [[' ' for _ in range(15)] for _ in range(15)]
player_x = 'X'
player_o = 'O'
def print_board():
for row in chess_board:
print(' '.join(row))
def check_win(board, player):
# 检查一行、一列和对角线是否有五个连续的棋子
for i in range(15):
if board[i] == [player] * 5:
return True
if (board[0][i], board[1][i], board[2][i],
board[7][i], board[8][i]) == [player] * 5:
return True
if (board[i][0], board[i][1], board[i][2],
board[i][7], board[i][8]) == [player] * 5:
return True
return False
def make_move(player, move):
x, y = move
chess_board[x][y] = player
def game_loop():
start_time = time.time()
while not check_win(chess_board, player_x) and not check_win(chess_board, player_o):
print_board()
print(f"Player {player_x}'s turn")
x, y = input("Enter your move (x, y): ").split(',')
x, y = int(x), int(y)
make_move(player_x, (x, y))
if check_win(chess_board, player_x):
break
player_x, player_o = player_o, player_x
end_time = time.time()
elapsed_time = end_time - start_time
print(f"Game over! Player {player_x} wins in {elapsed_time:.2f} seconds.")
game_loop()
python五子棋小游戏包括计时、得分、输赢的代码
在Python中实现五子棋小游戏通常涉及一些核心功能,如计时、得分计算和判断输赢条件。下面是一个简单的概述以及部分关键代码片段:
首先,你需要导入必要的库,例如`time`用于计时,`random`用于随机选择棋子颜色,以及一个二维数组或者列表来表示棋盘。
```python
import time
import random
# 定义棋盘大小和初始状态
board = [[' ' for _ in range(15)] for _ in range(15)]
```
计时可以使用`time.time()`函数开始和结束游戏,然后计算差值。例如:
```python
start_time = time.time()
# ...游戏循环...
game_duration = time.time() - start_time
print(f"游戏时间: {game_duration}秒")
```
得分通常会有一方不断增加,你可以创建两个变量分别代表双方得分:
```python
player_score = {'X': 0, 'O': 0}
```
对于判断输赢,你需要遍历棋盘,检查是否有连续五个同色的棋子。这里给出一个简化的判断方法:
```python
def check_win(player):
# 检查行、列和对角线
for direction in [(0, 1), (1, 0), (0, -1), (1, 1), (-1, 1), (-1, -1)]:
count = 0
for i in range(4):
if board[i][i + direction[0]] == player and \
board[i + 1][i + direction[1]] == player and \
board[i + 2][i + 2 * direction[0]] == player and \
board[i + 3][i + 3 * direction[1]] == player and \
board[i + 4][i + 4 * direction[0]] == player:
return True
return False
```
在每轮玩家落子之后,更新分数并检查是否有人获胜。完整的循环可能会包含类似这样的结构:
```python
while not (check_win('X') or check_win('O')) and not is_board_full(board):
# 让玩家交替下棋...
next_player = 'X' if current_player == 'O' else 'O'
place_piece(next_player)
current_player = next_player
if check_win(current_player):
print(f"{current_player} 获胜!")
else:
print("平局!")
```
阅读全文
相关推荐
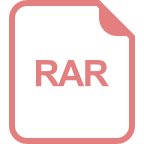
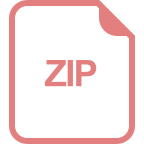
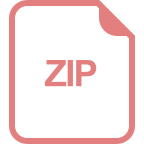
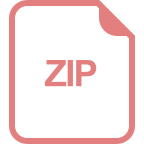
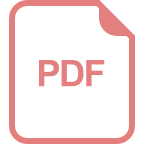
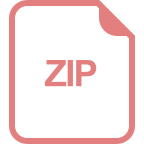
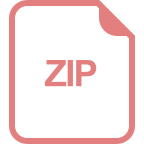
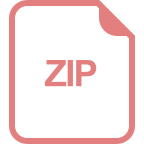
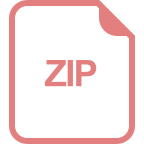
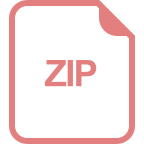
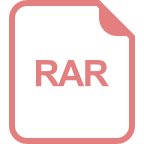
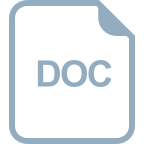