芯片为stm32f407,写一段串口3接收数据的中断函数
时间: 2023-12-06 21:31:53 浏览: 79
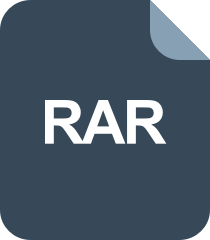
STM32f407串口中断函数
由于缺乏具体的需求和语言限制,本文将提供一个基于C++和CSGL库的简单的3D地形绘制示例程序。该程序将读取一个高程数据的DEM文件,并将其转换为一个网格模型,然后使用CSGL库来显示该模型。
首先,需要下载和安装CSGL库。在编写程序前,请确保已经熟悉了C++编程语言和OpenGL的基础知识。
以下是一个简单的程序示例:
```cpp
#include <iostream>
#include <fstream>
#include <cstring>
#include <cstdlib>
#include <csgl/csgl.h>
using namespace std;
const int MAX_VERTEX = 1000000; // 顶点数上限
const int MAX_FACE = 1000000; // 面数上限
struct Point {
float x, y, z;
};
struct Face {
int a, b, c;
};
int main(int argc, char **argv) {
if (argc != 2) {
cout << "Usage: " << argv[0] << " <dem_file>" << endl;
return 1;
}
// 读取DEM文件
ifstream fin(argv[1]);
if (!fin.good()) {
cout << "Failed to read file: " << argv[1] << endl;
return 1;
}
// 获取DEM尺寸
int width, height;
fin >> width >> height;
// 读取DEM数据
float *data = new float[width * height];
for (int i = 0; i < width * height; i++) {
fin >> data[i];
}
// 生成顶点和面
Point *vertices = new Point[width * height];
Face *faces = new Face[(width - 1) * (height - 1) * 2];
int vertexCount = 0;
int faceCount = 0;
for (int j = 0; j < height; j++) {
for (int i = 0; i < width; i++) {
vertices[vertexCount].x = i;
vertices[vertexCount].y = j;
vertices[vertexCount].z = data[j * width + i];
vertexCount++;
if (i < width - 1 && j < height - 1) {
faces[faceCount].a = j * width + i;
faces[faceCount].b = j * width + i + 1;
faces[faceCount].c = (j + 1) * width + i + 1;
faceCount++;
faces[faceCount].a = j * width + i;
faces[faceCount].b = (j + 1) * width + i + 1;
faces[faceCount].c = (j + 1) * width + i;
faceCount++;
}
}
}
// 释放DEM数据内存
delete[] data;
// 创建模型
CSGLModel *model = csglModelCreate();
model->numVertices = vertexCount;
model->vertices = new float[vertexCount * 3];
memcpy(model->vertices, vertices, vertexCount * sizeof(Point));
model->numFaces = faceCount;
model->faces = new int[faceCount * 3];
for (int i = 0; i < faceCount; i++) {
model->faces[i * 3] = faces[i].a;
model->faces[i * 3 + 1] = faces[i].b;
model->faces[i * 3 + 2] = faces[i].c;
}
// 释放顶点和面内存
delete[] vertices;
delete[] faces;
// 初始化CSGL库
csglInit(argc, argv);
// 创建窗口
csglCreateWindow("3D Terrain", 800, 600);
// 设置投影矩阵
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(75.0, 800.0 / 600.0, 0.1, 1000.0);
// 设置模型视图矩阵
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(0.0, 0.0, 10.0, 0.0, 0.0, 0.0, 0.0, 1.0, 0.0);
// 设置光照
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
GLfloat lightPos[] = {0.0, 0.0, 1.0, 0.0};
glLightfv(GL_LIGHT0, GL_POSITION, lightPos);
// 设置材质
GLfloat matAmbient[] = {0.5, 0.5, 0.5, 1.0};
GLfloat matDiffuse[] = {0.8, 0.8, 0.8, 1.0};
GLfloat matSpecular[] = {1.0, 1.0, 1.0, 1.0};
GLfloat matShininess[] = {100.0};
glMaterialfv(GL_FRONT_AND_BACK, GL_AMBIENT, matAmbient);
glMaterialfv(GL_FRONT_AND_BACK, GL_DIFFUSE, matDiffuse);
glMaterialfv(GL_FRONT_AND_BACK, GL_SPECULAR, matSpecular);
glMaterialfv(GL_FRONT_AND_BACK, GL_SHININESS, matShininess);
// 显示模型
csglDisplayModel(model);
// 进入消息循环
csglMainLoop();
// 释放模型内存
csglModelDestroy(model);
return 0;
}
```
该程序将使用命令行参数来指定输入的DEM文件。程序将读取DEM数据并转换为一个网格模型,然后使用CSGL库来显示该模型。
在主函数中,程序首先检查命令行参数的数量。如果参数数量不正确,则打印用法信息并返回错误代码。然后,程序打开指定的DEM文件并读取其尺寸和数据。接下来,程序将生成顶点和面,并使用CSGL库来创建一个模型对象。
在初始化CSGL库后,程序将创建一个窗口并设置投影矩阵和模型视图矩阵。程序还将启用光照和设置材质。最后,程序将显示模型并进入消息循环,直到用户关闭窗口。在退出循环后,程序将释放模型内存并返回0。
该程序仅仅是一个简单的示例,可以根据具体需求进行修改和扩展。例如,可以添加用户交互功能,使用户能够旋转和缩放模型。可以使用更复杂的材质和光照设置来增强模型的真实感。可以使用其他库来读取DEM数据,例如GDAL或OpenCV。
阅读全文
相关推荐
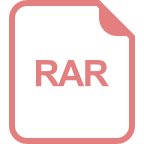

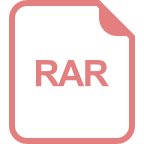
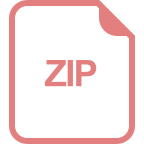
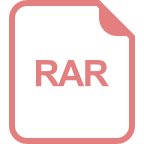
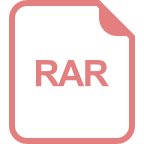
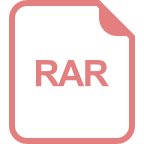
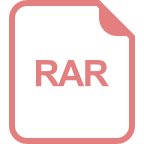
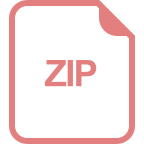
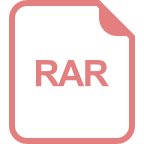
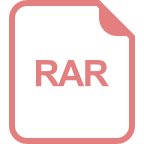
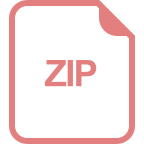
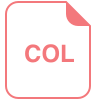
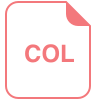
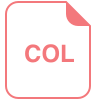
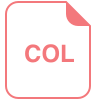