You are required to write a C program to: • Initialize GPIO peripherals • Initialise UART peripheral for receiving ASCII characters ‘A’ to ‘Z’ at baud 9600 • Initialise an internal array to hold 10 characters with head and tail: CharBuff • Repeat the following:o When data is received on the serial communication port, read ASCII character X, o If received character X is a capital letter add it to CharBuff, else ignore. o While CharBuff is not empty, transmit the morse code of the oldest stored character by blinking the LED (code provided for you). o When CharBuff is full, disable UART RX. o If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect your programme. You are recommended to use interrupt to control UART receiving data and coordinate the operation between CharBuff and P_LD2. 其中,启用uart代码位uart_enable();
时间: 2024-02-14 12:05:38 浏览: 165
以下是一个简单的示例程序,演示了如何实现上述要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include "stm32f4xx.h"
#define BUF_SIZE 10
// 初始化 GPIO
void init_gpio() {
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5; // LD2
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0; // P_B1
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
// 初始化 UART
void init_uart() {
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx;
USART_Init(USART2, &USART_InitStructure);
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
USART_Cmd(USART2, ENABLE);
}
// 定义 morse 码表
const char* morse_table[] = {
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-",
".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-",
".--", "-..-", "-.--", "--.."
};
// 定义字符缓冲区
char char_buff[BUF_SIZE];
int head = 0;
int tail = 0;
int count = 0;
int uart_enabled = 1;
// 将一个大写字母转换为 morse 码
void blink_morse(char c) {
if (c >= 'A' && c <= 'Z') {
const char* morse = morse_table[c - 'A'];
for (int i = 0; morse[i] != '\0'; i++) {
if (morse[i] == '.') {
GPIO_SetBits(GPIOA, GPIO_Pin_5); // LD2 on
delay(100);
GPIO_ResetBits(GPIOA, GPIO_Pin_5); // LD2 off
delay(100);
} else if (morse[i] == '-') {
GPIO_SetBits(GPIOA, GPIO_Pin_5); // LD2 on
delay(300);
GPIO_ResetBits(GPIOA, GPIO_Pin_5); // LD2 off
delay(100);
}
}
delay(200);
}
}
// 中断处理函数,当有数据接收时调用
void USART2_IRQHandler(void) {
if (USART_GetITStatus(USART2, USART_IT_RXNE) != RESET) {
char c = USART_ReceiveData(USART2);
if (c >= 'A' && c <= 'Z') {
if (count < BUF_SIZE) {
char_buff[tail] = c;
tail = (tail + 1) % BUF_SIZE;
count++;
if (count == BUF_SIZE) {
uart_enabled = 0;
USART_ITConfig(USART2, USART_IT_RXNE, DISABLE);
}
}
}
}
}
int main(void) {
init_gpio();
init_uart();
while (1) {
if (!uart_enabled && GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET) {
// 如果 UART RX 被禁用并且 P_B1 被按下,则重新启用 UART RX
head = 0;
tail = 0;
count = 0;
uart_enabled = 1;
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
}
if (count > 0) {
blink_morse(char_buff[head]);
head = (head + 1) % BUF_SIZE;
count--;
}
}
return 0;
}
```
这段代码使用 STM32F4 系列芯片作为开发板,通过 GPIO 控制板载 LED(LD2)的闪烁来输出 Morse 电码,通过 UART 串口接收来自外部设备的 ASCII 字符,将收到的大写字母存储在内部缓冲区(CharBuff)中,并在收到每个字符后立即将其转换为 Morse 电码输出。如果缓冲区已满,则禁用 UART 接收,并在按下按钮后重新启用它。
阅读全文
相关推荐
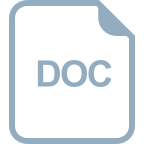
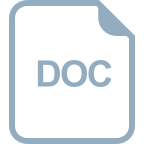
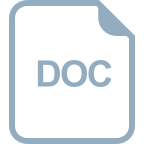


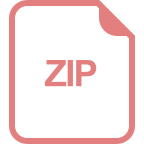
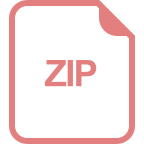
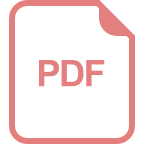
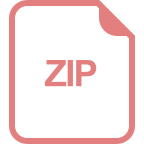
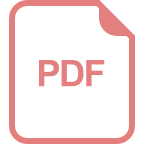
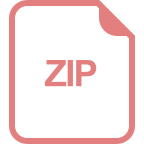
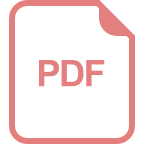
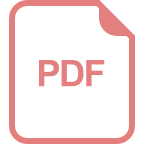
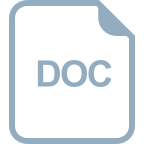
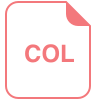

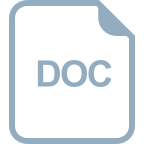
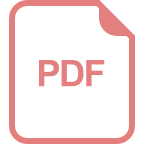