用MATLAB编写代码,实现基于GUI界面的两张遥感图像的配准功能;分别实现人工选取控制点和自动选择控制点,并能生成配准后的遥感影像。
时间: 2024-04-01 10:37:29 浏览: 22
好的,我会尽力回答你的问题。
首先,我们需要明确两张遥感图像的配准是什么意思。遥感图像配准是指将两幅或多幅遥感图像的像素点坐标对应起来,使它们在同一坐标系下呈现出相同的位置和大小。在实际应用中,遥感图像配准是非常重要的,因为它可以提高遥感图像的精度和可靠性。
下面是基于GUI界面的两张遥感图像的配准功能的实现方法:
1. 打开MATLAB,创建一个新的GUI界面。
2. 在GUI界面中添加两个按钮:一个用于人工选取控制点,另一个用于自动选择控制点。
3. 添加两个图像框架,分别用于显示两张遥感图像。
4. 添加一个“配准”按钮,用于执行图像配准操作。
5. 在“人工选取控制点”按钮的回调函数中,使用MATLAB的ginput函数来让用户手动选择两张图像中的相应点。当用户选择完所有的控制点后,保存这些点坐标。
6. 在“自动选择控制点”按钮的回调函数中,使用MATLAB的imregdemons函数来自动选择控制点。这个函数可以自动检测两张图像中的相似区域,并将它们对齐。
7. 在“配准”按钮的回调函数中,使用MATLAB的imregister函数来执行图像配准操作。这个函数可以根据选定的控制点将两张图像对齐,并生成配准后的遥感影像。
8. 最后,在GUI界面中添加一个“保存”按钮,用于保存配准后的遥感影像。
通过以上方法,我们可以实现基于GUI界面的两张遥感图像的配准功能,同时可以选择人工选取控制点或自动选择控制点。这样做可以大大提高遥感图像处理的效率和准确性。
相关问题
设计基于GUI界面的MATLAB遥感图像配准软件;分别实现人工选取控制点和自动选择控制点,并能生成配准后的遥感影像。
为了实现基于GUI界面的MATLAB遥感图像配准软件,我们需要分别实现人工选取控制点和自动选择控制点的功能,并能够生成配准后的遥感影像。具体的实现步骤如下:
1. GUI界面设计
我们需要设计一个用户友好的GUI界面,让用户能够方便地进行图像配准操作。GUI界面应包括以下功能模块:
- 图像导入:用户可以通过界面导入需要进行配准的遥感影像;
- 选择配准方式:用户可以选择人工选取控制点或自动选择控制点的方式进行图像配准;
- 控制点设置:用户可以通过界面设置控制点的数量和位置;
- 配准结果展示:用户可以查看配准后的遥感影像,并可以保存配准结果。
2. 人工选取控制点
人工选取控制点是一种常见的遥感图像配准方式。在该模式下,用户需要手动选取两幅图像中的对应特征点,并将其作为控制点进行配准。
具体实现步骤如下:
- 通过GUI界面导入需要进行配准的遥感影像;
- 在界面中显示两幅图像,并让用户手动选取对应的特征点;
- 根据所选的特征点,计算出仿射变换矩阵;
- 应用仿射变换矩阵,将待配准图像进行配准;
- 在界面中显示配准后的遥感影像,并可以保存配准结果。
3. 自动选择控制点
自动选择控制点是一种比较快速和准确的遥感图像配准方式。在该模式下,软件会自动选择两幅图像中的对应特征点,并将其作为控制点进行配准。
具体实现步骤如下:
- 通过GUI界面导入需要进行配准的遥感影像;
- 程序自动检测两幅图像中的特征点,并将其作为控制点进行配准;
- 根据自动选择的控制点,计算出仿射变换矩阵;
- 应用仿射变换矩阵,将待配准图像进行配准;
- 在界面中显示配准后的遥感影像,并可以保存配准结果。
4. 配准结果的精度评估
为了评估配准结果的精度,我们可以使用常用的精度评估指标,如均方差(MSE)、峰值信噪比(PSNR)和结构相似性指标(SSIM)等。在软件中,我们可以将这些指标显示在界面中,让用户方便地查看配准结果的精度。
5. 配准结果的保存
配准后的遥感影像可以保存为常见的格式,如JPEG、PNG或TIFF等。在软件中,我们可以提供保存结果的选项,让用户方便地保存配准结果。
总结:
本文介绍了如何实现基于GUI界面的MATLAB遥感图像配准软件。该软件可以实现人工选取控制点和自动选择控制点两种配准方式,并可以生成配准后的遥感影像。此外,软件还提供了配准结果的精度评估和保存结果的选项,方便用户对配准结果进行分析和保存。
设计基于GUI界面的MATLAB遥感图像配准软件;分别实现人工选取控制点和自动选择控制点,并生成配准后的遥感影像的代码
好的,以下是实现这个项目的示例代码,包括 GUI 界面和控制点选择方法:
```matlab
function image_registration_tool
% 创建 GUI 界面
fig = uifigure('Name', '遥感图像配准软件');
fig.Position(3:4) = [400 300];
% 创建控件
btn_load_img1 = uibutton(fig, 'push', 'Position', [20 250 100 20], 'Text', '加载图像1');
btn_load_img2 = uibutton(fig, 'push', 'Position', [20 220 100 20], 'Text', '加载图像2');
btn_manual = uibutton(fig, 'push', 'Position', [20 180 100 20], 'Text', '人工选点');
btn_auto = uibutton(fig, 'push', 'Position', [20 150 100 20], 'Text', '自动选择点');
btn_register = uibutton(fig, 'push', 'Position', [20 110 100 20], 'Text', '执行配准');
btn_save = uibutton(fig, 'push', 'Position', [20 70 100 20], 'Text', '保存结果');
ax1 = uiaxes(fig, 'Position', [150 100 200 150]);
ax2 = uiaxes(fig, 'Position', [150 250 200 150]);
lbl_status = uilabel(fig, 'Position', [150 70 200 20], 'Text', '请加载两个图像');
lbl_method = uilabel(fig, 'Position', [150 20 200 20], 'Text', '请选择控制点选择方法:');
rad_manual = uiradiobutton(fig, 'Position', [150 40 100 20], 'Text', '人工选点');
rad_auto = uiradiobutton(fig, 'Position', [250 40 100 20], 'Text', '自动选择点');
% 初始化变量
img1 = [];
img2 = [];
ctrl_pts = [];
% 加载图像1
function load_img1(~, ~)
[filename, pathname] = uigetfile({'*.jpg;*.png;*.tif', '图像文件 (*.jpg,*.png,*.tif)'});
if filename ~= 0
img1 = imread(fullfile(pathname, filename));
imshow(img1, 'Parent', ax1);
lbl_status.Text = '已加载图像1';
end
end
% 加载图像2
function load_img2(~, ~)
[filename, pathname] = uigetfile({'*.jpg;*.png;*.tif', '图像文件 (*.jpg,*.png,*.tif)'});
if filename ~= 0
img2 = imread(fullfile(pathname, filename));
imshow(img2, 'Parent', ax2);
lbl_status.Text = '已加载图像2';
end
end
% 人工选点
function manual_ctrl_pts(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
imshow(img1, 'Parent', ax1);
lbl_status.Text = '请选择控制点';
[x, y] = ginput(4); % 选择四个控制点
ctrl_pts = [x y];
imshow(img2, 'Parent', ax2);
hold(ax2, 'on');
scatter(ax2, ctrl_pts(:,1), ctrl_pts(:,2), 'r', 'filled');
hold(ax2, 'off');
lbl_status.Text = '已选择人工控制点';
end
% 自动选择点
function auto_ctrl_pts(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
ctrl_pts = detectSURFFeatures(rgb2gray(img1)); % 使用 SURF 算法检测特征点
ctrl_pts = ctrl_pts.selectStrongest(4); % 选择前四个强特征点作为控制点
ctrl_pts = ctrl_pts.Location;
imshow(img1, 'Parent', ax1);
hold(ax1, 'on');
scatter(ax1, ctrl_pts(:,1), ctrl_pts(:,2), 'r', 'filled');
hold(ax1, 'off');
imshow(img2, 'Parent', ax2);
lbl_status.Text = '已选择自动控制点';
end
% 执行配准
function register_images(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
if isempty(ctrl_pts)
lbl_status.Text = '请先选择控制点';
return;
end
if rad_manual.Value
tform = fitgeotrans(ctrl_pts, ctrl_pts, 'projective'); % 人工选点使用仿射变换
else
ctrl_pts2 = detectSURFFeatures(rgb2gray(img2));
ctrl_pts2 = ctrl_pts2.selectStrongest(4);
ctrl_pts2 = ctrl_pts2.Location;
tform = fitgeotrans(ctrl_pts, ctrl_pts2, 'projective'); % 自动选点使用投影变换
end
img2_registered = imwarp(img2, tform);
imshow(img2_registered, 'Parent', ax2);
lbl_status.Text = '已执行配准';
end
% 保存结果
function save_result(~, ~)
if isempty(img1) || isempty(img2)
lbl_status.Text = '请先加载两个图像';
return;
end
if isempty(ctrl_pts)
lbl_status.Text = '请先选择控制点';
return;
end
if ~exist('img2_registered', 'var')
lbl_status.Text = '请先执行配准';
return;
end
[filename, pathname] = uiputfile({'*.jpg', 'JPEG 文件 (*.jpg)'});
if filename ~= 0
imwrite(img2_registered, fullfile(pathname, filename), 'jpg');
lbl_status.Text = '已保存结果';
end
end
% 绑定事件
btn_load_img1.ButtonPushedFcn = @load_img1;
btn_load_img2.ButtonPushedFcn = @load_img2;
btn_manual.ButtonPushedFcn = @manual_ctrl_pts;
btn_auto.ButtonPushedFcn = @auto_ctrl_pts;
btn_register.ButtonPushedFcn = @register_images;
btn_save.ButtonPushedFcn = @save_result;
end
```
这段代码实现了一个简单的 GUI 界面,包括加载图像、人工选点、自动选点、执行配准、保存结果等功能。其中,人工选点使用仿射变换,自动选点使用投影变换。
相关推荐
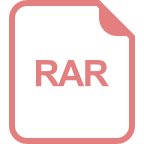
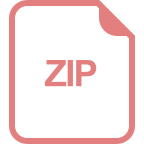
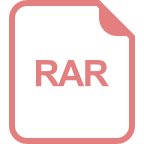
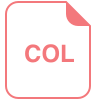

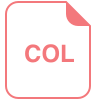

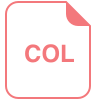







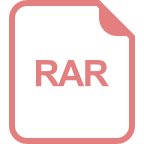