实现图像增强的直方图均衡 c++
时间: 2023-06-01 19:02:57 浏览: 42
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_PIXEL 255
#define MIN_PIXEL 0
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s input_image output_image\n", argv[0]);
return 1;
}
FILE *input_file = fopen(argv[1], "rb");
FILE *output_file = fopen(argv[2], "wb");
if (input_file == NULL || output_file == NULL) {
printf("Error: Could not open file\n");
return 1;
}
//读取位图文件头,位图信息头,颜色表
unsigned char bmp_header[54];
fread(bmp_header, sizeof(unsigned char), 54, input_file);
//获取位图宽度,高度,每个像素的颜色位数
int width = *(int*)&bmp_header[18];
int height = *(int*)&bmp_header[22];
int color_depth = *(int*)&bmp_header[28];
//计算每行像素的字节数
int row_size = width * color_depth / 8;
if (row_size % 4 != 0) {
row_size += 4 - (row_size % 4);
}
//读取像素数据
unsigned char *image_data = (unsigned char*)malloc(row_size * height);
fread(image_data, sizeof(unsigned char), row_size * height, input_file);
//计算直方图
int histogram[MAX_PIXEL + 1] = {0};
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
int index = i * row_size + j * color_depth / 8;
int pixel = image_data[index];
histogram[pixel]++;
}
}
//计算累积分布函数
int cdf[MAX_PIXEL + 1] = {0};
cdf[0] = histogram[0];
for (int i = 1; i <= MAX_PIXEL; i++) {
cdf[i] = cdf[i - 1] + histogram[i];
}
//计算像素值映射表
int map[MAX_PIXEL + 1] = {0};
float scale_factor = (float)(MAX_PIXEL - MIN_PIXEL) / (width * height);
for (int i = 0; i <= MAX_PIXEL; i++) {
map[i] = round((cdf[i] - cdf[0]) * scale_factor);
}
//应用像素值映射表
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
int index = i * row_size + j * color_depth / 8;
int pixel = image_data[index];
int new_pixel = map[pixel];
image_data[index] = new_pixel;
}
}
//写入位图文件头,位图信息头,颜色表
fwrite(bmp_header, sizeof(unsigned char), 54, output_file);
//写入像素数据
fwrite(image_data, sizeof(unsigned char), row_size * height, output_file);
//释放内存
free(image_data);
//关闭文件
fclose(input_file);
fclose(output_file);
return 0;
}
相关推荐
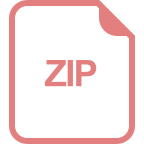
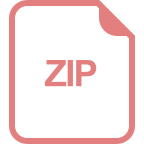
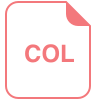
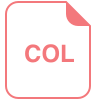
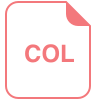
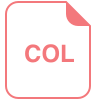
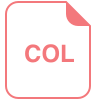









