用shell写一个遍历日期的脚本
时间: 2023-12-07 13:05:59 浏览: 38
以下是一个遍历日期的Shell脚本,从2021年1月1日到2021年12月31日:
```bash
#!/bin/bash
start_date="2021-01-01"
end_date="2021-12-31"
current="$start_date"
while [ "$current" != "$end_date" ]; do
echo "$current"
current=$(date -I -d "$current + 1 day")
done
echo "$current" # 打印最后一个日期
```
解释一下脚本的实现:
- `start_date` 和 `end_date` 定义了遍历日期的起始和终止日期。
- `current` 变量用于保存当前遍历到的日期,初始值为起始日期。
- `while` 循环条件中,如果当前日期不等于终止日期,则继续往下遍历。
- 在循环体中,使用 `echo` 打印当前日期。
- 最后,打印一下最后一个日期。
运行脚本后,会输出从2021年1月1日到2021年12月31日的所有日期。
相关问题
使用shell脚本写一个二叉树遍历
使用Shell脚本编写二叉树遍历可以通过递归的方式实现。下面是一个示例代码:
```shell
#!/bin/bash
# 定义二叉树节点结构
struct Node {
int data;
struct Node* left;
struct Node* right;
};
# 递归前序遍历二叉树
preorder() {
local node=$1
if [ -z "$node" ]; then
return
fi
echo $node->data
preorder $node->left
preorder $node->right
}
# 递归中序遍历二叉树
inorder() {
local node=$1
if [ -z "$node" ]; then
return
fi
inorder $node->left
echo $node->data
inorder $node->right
}
# 递归后序遍历二叉树
postorder() {
local node=$1
if [ -z "$node" ]; then
return
fi
postorder $node->left
postorder $node->right
echo $node->data
}
# 创建二叉树节点
createNode() {
local data=$1
local left=$2
local right=$3
local node="Node"
node+=" data=$data"
node+=" left=$left"
node+=" right=$right"
echo $node
}
# 构建一个示例二叉树
buildTree() {
local node1=$(createNode 1 null null)
local node2=$(createNode 2 null null)
local node3=$(createNode 3 null null)
local node4=$(createNode 4 null null)
local node5=$(createNode 5 null null)
local node6=$(createNode 6 null null)
local node7=$(createNode 7 null null)
node1+=" left=$node2"
node1+=" right=$node3"
node2+=" left=$node4"
node2+=" right=$node5"
node3+=" left=$node6"
node3+=" right=$node7"
echo $node1
}
# 主函数
main() {
local root=$(buildTree)
echo "前序遍历结果:"
preorder $root
echo "中序遍历结果:"
inorder $root
echo "后序遍历结果:"
postorder $root
}
# 调用主函数
main
```
这个示例代码中,我们定义了一个二叉树节点结构,并实现了递归的前序、中序和后序遍历函数。然后通过创建节点和构建二叉树的函数来构建一个示例二叉树。最后在主函数中调用遍历函数来输出遍历结果。
shell脚本怎么循环遍历一个数组
可以使用 for 循环来遍历一个数组,示例代码如下:
```shell
#!/bin/bash
# 定义一个数组
arr=("apple" "banana" "orange" "grape")
# 循环遍历数组
for item in ${arr[@]}
do
echo $item
done
```
输出结果为:
```
apple
banana
orange
grape
```
相关推荐
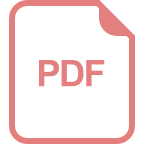
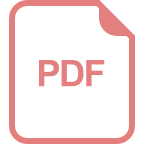
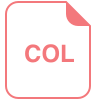
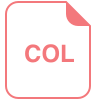
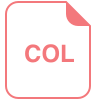
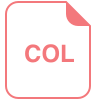
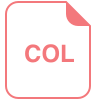







