Explain how Looper and Handler work together for threads execution in Android.
时间: 2023-11-28 21:47:26 浏览: 87
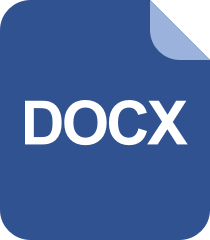
Handler和looper详解
In Android, Looper and Handler work together to execute tasks on a separate thread, also known as a background thread.
A Looper is an object that allows a thread to handle incoming messages or tasks. It runs in an infinite loop, waiting for new tasks to arrive. When a new task arrives, the Looper dispatches it to the appropriate Handler for processing.
A Handler is an object that receives tasks from the Looper and processes them. It is associated with a specific thread, and when a task is sent to a Handler, it is added to a message queue. The Handler processes the tasks one by one, in the order they were added to the queue.
Together, the Looper and Handler allow us to perform time-consuming tasks on a separate thread, without blocking the main thread. The main thread is responsible for handling UI events, and if it is blocked by a long-running task, the user interface will become unresponsive. By using Looper and Handler, we can keep the main thread free to handle UI events while executing time-consuming tasks on a separate thread.
Here is an example of how Looper and Handler work together:
```
public class MyThread extends Thread {
public Handler handler;
@Override
public void run() {
Looper.prepare();
handler = new Handler() {
@Override
public void handleMessage(Message msg) {
// Process the task here
}
};
Looper.loop();
}
}
```
In this example, we create a new thread called `MyThread`. In the `run()` method, we create a new `Looper` and call `prepare()` to initialize it. We then create a new `Handler` and associate it with the thread. Finally, we call `Looper.loop()` to start the infinite loop and wait for tasks to arrive.
To send a task to `MyThread`, we can use the `handler` object:
```
MyThread myThread = new MyThread();
myThread.start();
Message msg = myThread.handler.obtainMessage();
msg.obj = "Hello";
myThread.handler.sendMessage(msg);
```
In this example, we create a new message and set its `obj` property to `"Hello"`. We then send the message to `MyThread` using its `handler` object. When the message arrives, the `handleMessage()` method of the `Handler` will be called, and we can process the task there.
阅读全文
相关推荐
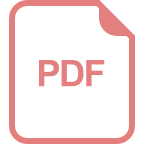
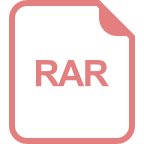
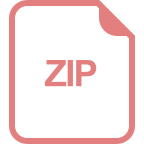
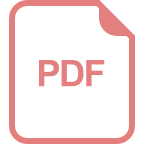
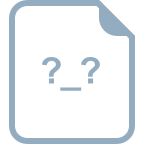
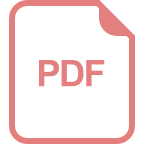
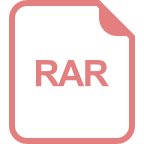
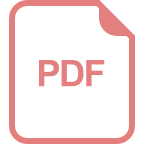
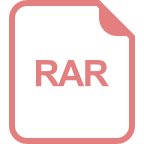
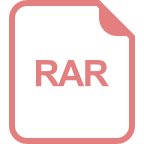