怎么修改这个错误 void CreateBTNode(BTNode *&,ElemType *)”: 无法将参数 2 从“const char [17]”转换为“ElemType *”
时间: 2024-04-29 08:21:31 浏览: 101
这个错误提示是因为你调用了函数 `CreateBTNode` 时,第二个参数类型不匹配。原本函数定义的参数类型是 `ElemType*`,但是你传入的是一个 `const char[17]` 类型的值。
要解决这个问题,你可以将第二个参数的类型修改为 `const char*`,或者将传入的参数类型改为 `ElemType*`。
示例代码如下:
```c++
void CreateBTNode(BTNode *&p, const char *data) {
// 这里可以将 const char* 类型的 data 转换为 ElemType* 类型
p = new BTNode;
p->data = new ElemType;
*(p->data) = *data;
p->lchild = p->rchild = NULL;
}
```
相关问题
void CreateBTree(BTNode*&,char*)无法将参数2从 const char[22]转换为char 怎么解决
这个错误通常发生在函数签名中,`BTNode*&` 和 `char*` 表示的参数类型不兼容,尤其是当你试图传递一个常量字符数组 `const char[22]` 给接受 `char*` 的参数时。因为 `const char[22]` 是常量指针,而 `char*` 可以指向非常量内存。
为了解决这个问题,你可以做以下几件事:
1. **修改函数参数**:如果允许输入的字符串是常量,可以将函数的第二个参数改为 `const char*` 或者 `const char[22]` 直接,这样就无需改变传入的值。
```cpp
void CreateBTree(BTNode*&, const char*);
```
2. **复制字符串**:如果需要修改字符串内容,可以在函数内部创建一个新的动态缓冲区来存储数据。
```cpp
void CreateBTree(BTNode*&, char (&temp)[22]) {
strcpy(temp, input);
}
```
这里假设 `input` 是你要传递的常量字符数组。
3. **使用 `std::string`**:如果你正在使用 C++,可以改用 `std::string` 类型作为参数,这样可以直接处理字符串,避免了指针类型的困扰。
```cpp
void CreateBTree(BTNode*&, std::string&);
```
然后传递 `std::string` 对象。
记得在实际应用中选择合适的方法,根据函数的具体需求和上下文。
请找出下列代码的问题并解决:#include<iostream> using namespace std; #define Maxsize 100 typedef struct node//二叉树结构 { char data; struct node* lchild; struct node* rchild; }BTnode; void CreateNode(BTnode*& bt) { char h; h = getchar(); if (h != '#') { bt = (BTnode*)malloc(sizeof(BTnode)); bt->data = h; CreateNode(bt->lchild); CreateNode(bt->rchild); } else bt = NULL; } void DestoryNode(BTnode*& bt) { if (bt != NULL) { DestoryNode(bt->lchild); DestoryNode(bt->rchild); free(bt); } } typedef struct//顺序队列 { BTnode* data[Maxsize]; int front; int rear; }SqQueue; typedef struct//顺序栈 { BTnode* data[Maxsize]; int top; }SqStack; void InitQueue(SqQueue*& q) { q = (SqQueue*)malloc(sizeof(SqQueue)); q->front = q->rear = -1; } void InitStack(SqStack*& s) { s = (SqStack*)malloc(sizeof(SqStack)); s->top = -1; } void DestoryQueue(SqQueue*& q) { free(q); } void DestoryStack(SqStack*& s) { free(s); } bool QueueEmpty(SqQueue* q) { return(q->front == q->rear); } bool StackEmpty(SqStack* s) { return(s->top == -1); } bool enQueue(SqQueue*& q, BTnode*& node) { if (q->rear == Maxsize - 1) return 0; q->rear++; q->data[q->rear] = node; return 1; } bool Push(SqStack*& s, BTnode*& node) { if (s->top == Maxsize - 1) return 0; s->top++; s->data[s->top] = node; return 1; } bool deQueue(SqQueue*& q, BTnode*& node) { if (q->front == q->rear) return 0; q->front++; node = q->data[q->front]; return 1; } bool Pop(SqStack*& s) { if (s->top == -1) return 0; cout << s->data[s->top] << "\t"; s->top--; return 1; } void LevelOrder(BTnode* bt) { BTnode* p; p = new BTnode; SqQueue* qu; SqStack* st; InitStack(st); InitQueue(qu); enQueue(qu, bt); while (!QueueEmpty(qu)) { deQueue(qu, p); Push(st, bt); if (p->lchild != NULL) enQueue(qu, p->lchild); if (p->rchild != NULL) enQueue(qu, p->rchild); } cout << "二叉树的自下而上,从右到左的层次遍历结果:" << endl; if (!StackEmpty(st)) Pop(st); DestoryQueue(qu); DestoryNode(p); DestoryStack(st); } int main() { BTnode* B; cout << "输入二叉树:" << endl; CreateNode(B); LevelOrder(B); DestoryNode(B); return 0; }
代码存在以下问题:
1. 在LevelOrder函数中,创建了一个新的BTnode指针p,但没有初始化,可能会引起未知的错误。
2. 在LevelOrder函数中,将二叉树节点指针p入栈时,使用了错误的变量bt,应该使用p。
3. 在LevelOrder函数中,调用Pop函数时,打印的是栈顶元素的data值,但实际上应该打印的是指针p指向的节点的data值。
修改后的代码如下:
```
#include<iostream>
using namespace std;
#define Maxsize 100
typedef struct node//二叉树结构
{
char data;
struct node* lchild;
struct node* rchild;
}BTnode;
void CreateNode(BTnode*& bt)
{
char h;
h = getchar();
if (h != '#')
{
bt = (BTnode*)malloc(sizeof(BTnode));
bt->data = h;
CreateNode(bt->lchild);
CreateNode(bt->rchild);
}
else bt = NULL;
}
void DestoryNode(BTnode*& bt)
{
if (bt != NULL)
{
DestoryNode(bt->lchild);
DestoryNode(bt->rchild);
free(bt);
}
}
typedef struct//顺序队列
{
BTnode* data[Maxsize];
int front;
int rear;
}SqQueue;
typedef struct//顺序栈
{
BTnode* data[Maxsize];
int top;
}SqStack;
void InitQueue(SqQueue*& q)
{
q = (SqQueue*)malloc(sizeof(SqQueue));
q->front = q->rear = -1;
}
void InitStack(SqStack*& s)
{
s = (SqStack*)malloc(sizeof(SqStack));
s->top = -1;
}
void DestoryQueue(SqQueue*& q)
{
free(q);
}
void DestoryStack(SqStack*& s)
{
free(s);
}
bool QueueEmpty(SqQueue* q)
{
return(q->front == q->rear);
}
bool StackEmpty(SqStack* s)
{
return(s->top == -1);
}
bool enQueue(SqQueue*& q, BTnode*& node)
{
if (q->rear == Maxsize - 1)
return 0;
q->rear++;
q->data[q->rear] = node;
return 1;
}
bool Push(SqStack*& s, BTnode*& node)
{
if (s->top == Maxsize - 1)
return 0;
s->top++;
s->data[s->top] = node;
return 1;
}
bool deQueue(SqQueue*& q, BTnode*& node)
{
if (q->front == q->rear)
return 0;
q->front++;
node = q->data[q->front];
return 1;
}
bool Pop(SqStack*& s)
{
if (s->top == -1)
return 0;
cout << s->data[s->top]->data << "\t";
s->top--;
return 1;
}
void LevelOrder(BTnode* bt)
{
BTnode* p = NULL;
SqQueue* qu;
SqStack* st;
InitStack(st);
InitQueue(qu);
enQueue(qu, bt);
while (!QueueEmpty(qu))
{
deQueue(qu, p);
Push(st, p);
if (p->lchild != NULL)
enQueue(qu, p->lchild);
if (p->rchild != NULL)
enQueue(qu, p->rchild);
}
cout << "二叉树的自下而上,从右到左的层次遍历结果:" << endl;
while (Pop(st));
DestoryQueue(qu);
DestoryStack(st);
}
int main()
{
BTnode* B;
cout << "输入二叉树:" << endl;
CreateNode(B);
LevelOrder(B);
DestoryNode(B);
return 0;
}
```
阅读全文
相关推荐
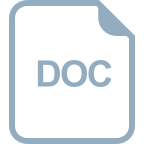
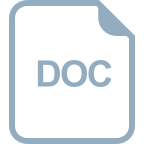
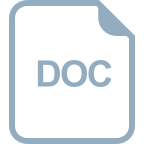













