rsa加密算法c语言实现
时间: 2023-09-23 17:11:34 浏览: 45
我可以为您提供RSA加密算法的C语言实现代码。以下是示例代码:
```
#include<stdio.h>
#include<math.h>
int p = 7; //素数p
int q = 17; //素数q
int e = 5; //加密密钥e
int d = 29; //解密密钥d
int n = 119; //p与q的乘积n
//欧几里得扩展算法
int euclid(int a, int b, int *x, int *y) {
if (b == 0) {
*x = 1;
*y = 0;
return a;
} else {
int r = euclid(b, a%b, y, x);
*y -= a/b * (*x);
return r;
}
}
//快速幂取模算法
int fast_power(int a, int b) {
int result = 1;
while (b != 0) {
if (b & 1 != 0) {
result = (result * a) % n;
}
a = (a * a) % n;
b = b >> 1;
}
return result;
}
//加密函数
int encrypt(int plaintext) {
int ciphertext = fast_power(plaintext, e);
return ciphertext;
}
//解密函数
int decrypt(int ciphertext) {
int plaintext = fast_power(ciphertext, d);
return plaintext;
}
int main() {
int plaintext = 65; //明文值
printf("明文:%d\n", plaintext);
int ciphertext = encrypt(plaintext); //加密
printf("密文:%d\n", ciphertext);
int recovered_plaintext = decrypt(ciphertext); //解密
printf("解密后的明文:%d\n", recovered_plaintext);
}
```
当然,这只是一个简单的示例。在实际应用中,RSA加密还需要考虑更多的因素,比如如何选择素数、如何生成密钥、如何保证安全性等等。如果您需要进一步了解,建议参考更加详细的教程或者专业的加密算法库。
相关推荐
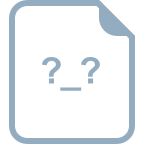
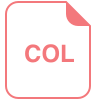
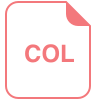
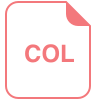
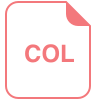
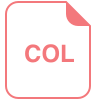








