使用python完成贪吃蛇游戏
时间: 2023-07-20 13:06:00 浏览: 41
好的,让我们开始吧!首先,我们需要安装Pygame库,它是一个非常流行的Python游戏开发库。
在命令行中输入以下命令安装Pygame:
```
pip install pygame
```
安装完成后,我们可以开始编写贪吃蛇游戏了。下面是完整的代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 游戏界面宽度和高度
width = 640
height = 480
# 创建游戏窗口
window = pygame.display.set_mode((width, height))
# 设置游戏标题
pygame.display.set_caption("贪吃蛇游戏")
# 贪吃蛇的颜色
snake_color = (0, 255, 0)
# 食物的颜色
food_color = (255, 0, 0)
# 贪吃蛇坐标和长度
snake = [(200, 200), (210, 200), (220, 200), (230, 200)]
snake_length = len(snake)
# 贪吃蛇移动的方向
direction = "right"
# 随机生成食物的位置
food_pos = (random.randint(0, width-10)//10*10, random.randint(0, height-10)//10*10)
# 得分
score = 0
# 加载字体
font = pygame.font.SysFont("Arial", 20)
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 关闭游戏
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
# 处理按键事件
if event.key == pygame.K_UP and direction != "down":
direction = "up"
elif event.key == pygame.K_DOWN and direction != "up":
direction = "down"
elif event.key == pygame.K_LEFT and direction != "right":
direction = "left"
elif event.key == pygame.K_RIGHT and direction != "left":
direction = "right"
# 移动贪吃蛇
if direction == "up":
snake.pop()
snake.insert(0, (snake[0][0], snake[0][1]-10))
elif direction == "down":
snake.pop()
snake.insert(0, (snake[0][0], snake[0][1]+10))
elif direction == "left":
snake.pop()
snake.insert(0, (snake[0][0]-10, snake[0][1]))
elif direction == "right":
snake.pop()
snake.insert(0, (snake[0][0]+10, snake[0][1]))
# 判断贪吃蛇是否吃到了食物
if snake[0] == food_pos:
# 重新生成食物的位置
food_pos = (random.randint(0, width-10)//10*10, random.randint(0, height-10)//10*10)
# 增加贪吃蛇的长度
snake.append(snake[-1])
# 得分加1
score += 1
# 检测贪吃蛇是否撞到墙壁或自己
if snake[0][0] < 0 or snake[0][0] > width-10 or snake[0][1] < 0 or snake[0][1] > height-10:
# 游戏结束
print("Game Over!")
pygame.quit()
exit()
for i in range(1, len(snake)):
if snake[0] == snake[i]:
# 游戏结束
print("Game Over!")
pygame.quit()
exit()
# 渲染游戏界面
window.fill((255, 255, 255))
for pos in snake:
pygame.draw.rect(window, snake_color, pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(window, food_color, pygame.Rect(food_pos[0], food_pos[1], 10, 10))
# 显示得分
text_surface = font.render("Score: "+str(score), True, (0, 0, 0))
window.blit(text_surface, (10, 10))
pygame.display.update()
# 控制游戏帧率
pygame.time.Clock().tick(10)
```
运行代码,就可以开始玩贪吃蛇游戏了!
相关推荐
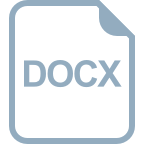
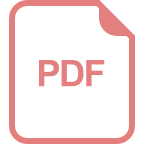
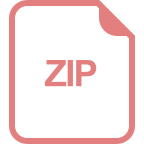









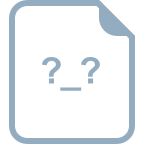
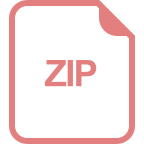
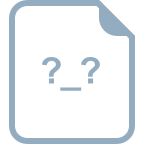