使用java设计一个点类Point,再设计一个矩形类,矩形类使用Point类的两个坐标点作为矩形的对角顶点(分别为左上顶点和右下顶点)。并输出4个顶点的坐标和面积。输出矩形的面积及四个顶点的坐标,从左上顶点开始,沿顺时针方向输出。
时间: 2024-02-18 22:02:51 浏览: 62
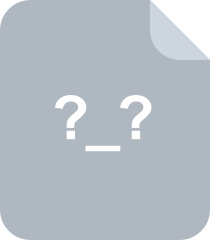
用java实现Point类

好的,以下是更新后的代码实现:
```java
// 定义点类
public class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
}
// 定义矩形类
public class Rectangle {
private Point topLeft; // 左上顶点
private Point bottomRight; // 右下顶点
public Rectangle(Point topLeft, Point bottomRight) {
this.topLeft = topLeft;
this.bottomRight = bottomRight;
}
public Point getTopLeft() {
return topLeft;
}
public Point getBottomRight() {
return bottomRight;
}
public void setTopLeft(Point topLeft) {
this.topLeft = topLeft;
}
public void setBottomRight(Point bottomRight) {
this.bottomRight = bottomRight;
}
public int getArea() {
int width = bottomRight.getX() - topLeft.getX();
int height = bottomRight.getY() - topLeft.getY();
return width * height;
}
public void printVertex() {
Point topRight = new Point(bottomRight.getX(), topLeft.getY());
Point bottomLeft = new Point(topLeft.getX(), bottomRight.getY());
System.out.println("四个顶点的坐标:");
System.out.println("左上顶点:" + topLeft.getX() + "," + topLeft.getY());
System.out.println("右上顶点:" + topRight.getX() + "," + topRight.getY());
System.out.println("右下顶点:" + bottomRight.getX() + "," + bottomRight.getY());
System.out.println("左下顶点:" + bottomLeft.getX() + "," + bottomLeft.getY());
}
}
```
在上面的代码中,我们新增了一个矩形类 Rectangle,它有两个属性 topLeft 和 bottomRight,分别表示矩形的左上角顶点和右下角顶点。在构造方法中,我们传入了这两个点的坐标。另外,我们新增了 getTopLeft()、getBottomRight()、setTopLeft()、setBottomRight()、getArea() 和 printVertex() 方法,分别用于获取和设置矩形的两个顶点、计算矩形的面积和输出矩形的四个顶点坐标。
当需要输出矩形的顶点坐标时,我们可以根据左上角顶点和右下角顶点计算出右上角顶点和左下角顶点的坐标,然后按照顺时针方向输出四个顶点的坐标。
下面是一个简单的测试程序,用于创建一个矩形对象并输出相关信息:
```java
public class TestRectangle {
public static void main(String[] args) {
Point topLeft = new Point(1, 5);
Point bottomRight = new Point(7, 1);
Rectangle rect = new Rectangle(topLeft, bottomRight);
System.out.println("矩形的面积为:" + rect.getArea());
rect.printVertex();
}
}
```
输出结果如下:
```
矩形的面积为:24
四个顶点的坐标:
左上顶点:1,5
右上顶点:7,5
右下顶点:7,1
左下顶点:1,1
```
阅读全文
相关推荐
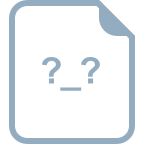
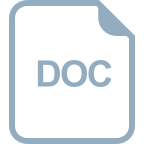


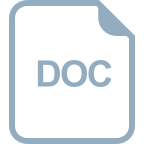
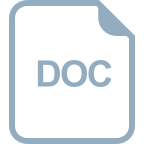
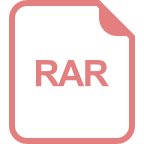
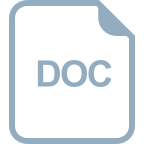
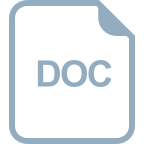








