封装一个图片审核的代码工具类,图片审核调用百度api内容审核功能,要求到处可用
时间: 2023-10-16 13:09:08 浏览: 89
好的,这里我给出一个 Python 版本的图片审核工具类,使用了百度的内容审核 API。注意,这里需要使用到百度的 API Key 和 Secret Key,请根据自己的实际情况进行替换。
```python
import requests
import base64
class ImageModeration:
def __init__(self, api_key, secret_key):
self.api_key = api_key
self.secret_key = secret_key
self.access_token = self._get_access_token()
def _get_access_token(self):
url = 'https://aip.baidubce.com/oauth/2.0/token'
params = {
'grant_type': 'client_credentials',
'client_id': self.api_key,
'client_secret': self.secret_key
}
response = requests.get(url, params=params).json()
return response['access_token']
def _image_to_base64(self, image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
base64_data = base64.b64encode(image_data)
return base64_data.decode('utf-8')
def _request(self, url, data):
headers = {'Content-Type': 'application/json'}
params = {'access_token': self.access_token}
response = requests.post(url, params=params, headers=headers, json=data).json()
return response
def image_moderation(self, image_path, image_type='BASE64', scenes=['antiporn'], threshold=0.95):
if image_type == 'BASE64':
image_data = self._image_to_base64(image_path)
else:
with open(image_path, 'rb') as f:
image_data = f.read()
url = f'https://aip.baidubce.com/rest/2.0/solution/v1/img_censor/v2/user_defined?access_token={self.access_token}'
data = {
'image': image_data,
'image_type': image_type,
'scenes': scenes,
'threshold': threshold
}
response = self._request(url, data)
return response
```
该工具类的使用方法如下:
```python
api_key = 'your_api_key'
secret_key = 'your_secret_key'
moderation = ImageModeration(api_key, secret_key)
result = moderation.image_moderation('your_image_path')
print(result)
```
其中,`api_key` 和 `secret_key` 分别为你的百度 API Key 和 Secret Key,`your_image_path` 为待审核的图片路径。`image_moderation` 方法的返回值是一个 JSON 对象,包含了审核结果的详细信息。你可以根据需要对这些信息进行解析和处理。
阅读全文
相关推荐
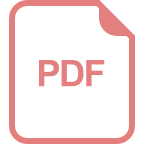
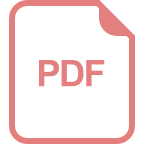
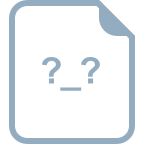
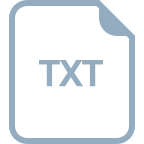
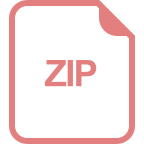
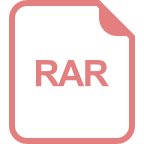
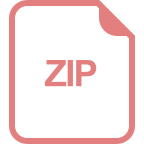
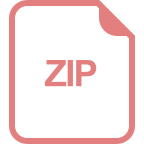
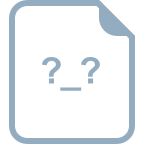
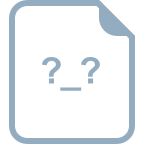
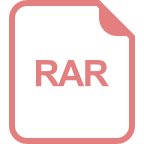
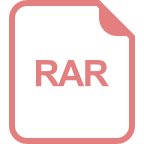
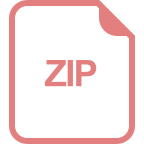
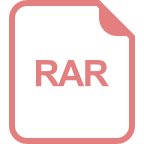
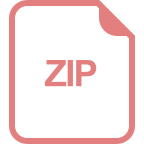
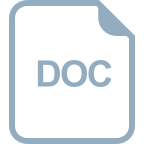
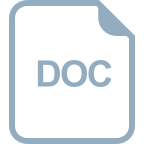
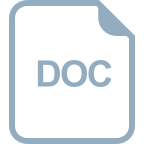