1.编写一个程序,实现JDK中ReadWriteLock的功能ReadWriteLock读写锁:读锁中的代码执行时,写锁中的代码被阻塞,写锁中的代码执行时,读锁中的代码也会被阻塞,也就是读锁和写锁会相互阻塞:但是读锁中的代码执行时,其他加读锁的代码不会受影响。
时间: 2024-03-03 19:47:54 浏览: 62
下面是一个简单的使用Java的ReentrantReadWriteLock类实现读写锁的示例代码:
```java
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private static final ReentrantReadWriteLock lock = new ReentrantReadWriteLock();
private static final String[] data = new String[10];
public static void main(String[] args) {
// 启动10个读线程
for (int i = 0; i < 10; i++) {
new Thread(new Reader()).start();
}
// 启动5个写线程
for (int i = 0; i < 5; i++) {
new Thread(new Writer(i)).start();
}
}
static class Reader implements Runnable {
@Override
public void run() {
while (true) {
lock.readLock().lock(); // 获取读锁
try {
// 读取数据
for (String s : data) {
System.out.println(Thread.currentThread().getName() + " read " + s);
}
} finally {
lock.readLock().unlock(); // 释放读锁
}
}
}
}
static class Writer implements Runnable {
private int index;
public Writer(int index) {
this.index = index;
}
@Override
public void run() {
while (true) {
lock.writeLock().lock(); // 获取写锁
try {
// 修改数据
data[index] = "data-" + index;
System.out.println(Thread.currentThread().getName() + " write " + data[index]);
} finally {
lock.writeLock().unlock(); // 释放写锁
}
}
}
}
}
```
在这个示例中,我们使用一个字符串数组作为共享数据,启动了10个读线程和5个写线程。读线程会重复地读取数据并输出,写线程会修改数据并输出。
在读线程中获取读锁,读取数据,然后释放读锁。在写线程中获取写锁,修改数据,然后释放写锁。由于读锁和写锁是互斥的,所以在写线程获取写锁时,读线程会被阻塞。同样地,在读线程获取读锁时,其他读线程不会受到影响。
阅读全文
相关推荐
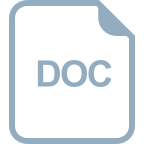
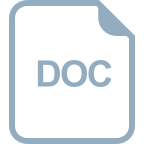
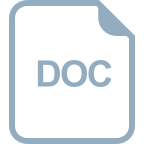



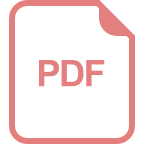
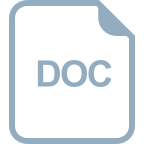
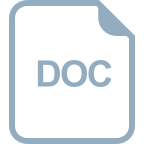
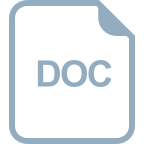
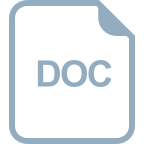
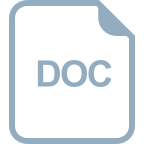
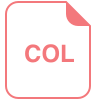
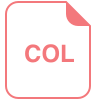
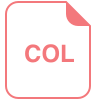
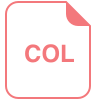
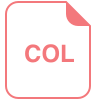
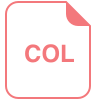
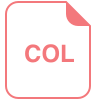