@RequestMapping("/systemChange") public String systemChange(String id) { Student student = studentService.getById(id); LambdaQueryWrapper<Student> studentLambdaQueryWrapper = new LambdaQueryWrapper<>(); studentLambdaQueryWrapper.eq(Student::getSex,student.getSex()); //班级的学生 if (!ObjectUtils.isEmpty(student.getGrade())){ studentLambdaQueryWrapper.eq(Student::getGrade,student.getGrade()); } //并且存在宿舍的 studentLambdaQueryWrapper.isNotNull(Student::getDormitoryId); List<Student> studentList = studentService.list(studentLambdaQueryWrapper); //查出自己的文件答案 LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>(); topicResultLambdaQueryWrapper.eq(TopicResult::getStuNum, student.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2)); List<TopicResult> topicResults = topicResultService.list(topicResultLambdaQueryWrapper); for (Student student1 : studentList) { LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper1 = new LambdaQueryWrapper<>(); topicResultLambdaQueryWrapper1.eq(TopicResult::getStuNum, student1.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2)); List<TopicResult> results = topicResultService.list(topicResultLambdaQueryWrapper1); if (topicResults.get(0).getOptionId().equals(results.get(0).getOptionId()) || topicResults.get(1).getOptionId().equals(results.get(1).getOptionId()) ){ Dormitory dormitory = dormitoryService.getById(student1.getDormitoryId()); if (dormitory.getNum()<dormitory.getTotal()){ student.setDormitoryId(dormitory.getId()); studentService.updateById(student); dormitory.setNum(dormitory.getNum()+1); dormitoryService.updateById(dormitory); return "redirect:list"; } } }请详细解释每一行代码
时间: 2024-03-14 10:48:18 浏览: 36
好的,我来详细解释一下这段代码的含义和作用。
```java
@RequestMapping("/systemChange")
public String systemChange(String id) {
// 获取指定 id 的学生信息
Student student = studentService.getById(id);
// 构建查询条件,查找与当前学生性别一致、班级一致且有宿舍的其他学生列表
LambdaQueryWrapper<Student> studentLambdaQueryWrapper = new LambdaQueryWrapper<>();
studentLambdaQueryWrapper.eq(Student::getSex,student.getSex());
if (!ObjectUtils.isEmpty(student.getGrade())){
studentLambdaQueryWrapper.eq(Student::getGrade,student.getGrade());
}
studentLambdaQueryWrapper.isNotNull(Student::getDormitoryId);
List<Student> studentList = studentService.list(studentLambdaQueryWrapper);
// 查找当前学生的题目答案
LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>();
topicResultLambdaQueryWrapper.eq(TopicResult::getStuNum, student.getStudentNumber())
.and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));
List<TopicResult> topicResults = topicResultService.list(topicResultLambdaQueryWrapper);
// 遍历其他学生列表,查找是否有与当前学生答案相同的学生
for (Student student1 : studentList) {
LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper1 = new LambdaQueryWrapper<>();
topicResultLambdaQueryWrapper1.eq(TopicResult::getStuNum, student1.getStudentNumber())
.and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));
List<TopicResult> results = topicResultService.list(topicResultLambdaQueryWrapper1);
if (topicResults.get(0).getOptionId().equals(results.get(0).getOptionId())
|| topicResults.get(1).getOptionId().equals(results.get(1).getOptionId())
){
// 如果有相同的学生,则将当前学生分配到该学生所在的宿舍中
Dormitory dormitory = dormitoryService.getById(student1.getDormitoryId());
if (dormitory.getNum()<dormitory.getTotal()){
student.setDormitoryId(dormitory.getId());
studentService.updateById(student);
dormitory.setNum(dormitory.getNum()+1);
dormitoryService.updateById(dormitory);
return "redirect:list";
}
}
}
}
```
具体解释如下:
1. `@RequestMapping("/systemChange")`:该注解指定了请求路径 `/systemChange`,当用户访问该路径时,该方法会被执行。
2. `public String systemChange(String id)`:该方法的参数 `id` 表示需要更换宿舍的学生的 id。
3. `Student student = studentService.getById(id);`:通过 `id` 获取需要更换宿舍的学生信息。
4. `LambdaQueryWrapper<Student> studentLambdaQueryWrapper = new LambdaQueryWrapper<>();`:使用 Lambda 表达式构建查询条件,用于查找与当前学生性别一致、班级一致且有宿舍的其他学生列表。
5. `studentLambdaQueryWrapper.eq(Student::getSex,student.getSex());`:添加查询条件,要求性别与当前学生一致。
6. `if (!ObjectUtils.isEmpty(student.getGrade())){ studentLambdaQueryWrapper.eq(Student::getGrade,student.getGrade()); }`:如果当前学生的班级不为空,则添加查询条件,要求班级与当前学生一致。
7. `studentLambdaQueryWrapper.isNotNull(Student::getDormitoryId);`:添加查询条件,要求有宿舍。
8. `List<Student> studentList = studentService.list(studentLambdaQueryWrapper);`:执行查询,得到符合条件的学生列表。
9. `LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>();`:使用 Lambda 表达式构建查询条件,用于查找当前学生的题目答案。
10. `topicResultLambdaQueryWrapper.eq(TopicResult::getStuNum, student.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));`:添加查询条件,要求学生学号等于当前学生的学号,并且题目编号为 1 或者 2。
11. `List<TopicResult> topicResults = topicResultService.list(topicResultLambdaQueryWrapper);`:执行查询,得到当前学生的题目答案。
12. `for (Student student1 : studentList) {`:遍历其他符合条件的学生列表。
13. `LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper1 = new LambdaQueryWrapper<>();`:使用 Lambda 表达式构建查询条件,用于查找当前学生的题目答案。
14. `topicResultLambdaQueryWrapper1.eq(TopicResult::getStuNum, student1.getStudentNumber()) .and(qw -> qw.eq(TopicResult::getTopicId, 1).or().eq(TopicResult::getTopicId, 2));`:添加查询条件,要求学生学号等于当前遍历到的学生的学号,并且题目编号为 1 或者 2。
15. `List<TopicResult> results = topicResultService.list(topicResultLambdaQueryWrapper1);`:执行查询,得到当前遍历到的学生的题目答案。
16. `if (topicResults.get(0).getOptionId().equals(results.get(0).getOptionId()) || topicResults.get(1).getOptionId().equals(results.get(1).getOptionId())){`:如果当前遍历到的学生的题目答案和当前学生的题目答案相同,则执行以下代码。
17. `Dormitory dormitory = dormitoryService.getById(student1.getDormitoryId());`:获取当前遍历到的学生所在的宿舍。
18. `if (dormitory.getNum()<dormitory.getTotal()){`:如果该宿舍还有空位,则执行以下代码。
19. `student.setDormitoryId(dormitory.getId());`:将当前学生分配到该宿舍中。
20. `studentService.updateById(student);`:更新当前学生的信息。
21. `dormitory.setNum(dormitory.getNum()+1);`:将该宿舍的已用床位数量加 1。
22. `dormitoryService.updateById(dormitory);`:更新该宿舍的信息。
23. `return "redirect:list";`:重定向到列表页面。
希望以上解释能够帮到您,如果您还有其他问题,可以继续问我。
相关推荐
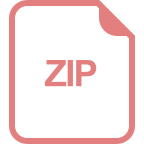















