python tkinter中控件根据屏幕大小缩放控件
时间: 2023-11-06 17:14:34 浏览: 1039
在Python的Tkinter中,可以使用以下方法让控件根据屏幕大小自适应缩放:
1. `place` 方法:可以使用相对或绝对的坐标来定位控件,通过改变控件的尺寸和位置,让控件随窗口大小改变而自适应缩放。
```python
import tkinter as tk
class App:
def __init__(self, master):
self.master = master
self.label = tk.Label(master, text="Hello World", font=("Arial", 12))
self.label.place(relx=0.5, rely=0.5, anchor='center')
# 使用相对坐标,将标签置于窗口中心
def resize(self, event):
w, h = event.width, event.height
size = int((w + h) / 30)
self.label.config(font=("Arial", size))
root = tk.Tk()
app = App(root)
root.geometry("400x300") # 设置窗口大小
root.bind("<Configure>", app.resize) # 监听窗口大小变化
root.mainloop()
```
在上面的例子中,我们使用 `place` 方法将标签置于窗口中心,然后使用 `resize` 方法监听窗口大小变化,然后根据窗口的宽度和高度计算出新的字体大小,并更新标签的字体。
2. `grid` 方法:可以使用行和列来定位控件,通过改变控件的尺寸和位置,让控件随窗口大小改变而自适应缩放。
```python
import tkinter as tk
class App:
def __init__(self, master):
self.master = master
self.label = tk.Label(master, text="Hello World", font=("Arial", 12))
self.label.grid(row=0, column=0)
# 将标签置于第一行第一列
def resize(self, event):
w, h = event.width, event.height
size = int((w + h) / 30)
self.label.config(font=("Arial", size))
root = tk.Tk()
app = App(root)
root.geometry("400x300") # 设置窗口大小
root.columnconfigure(0, weight=1) # 列0随窗口大小改变而自适应
root.rowconfigure(0, weight=1) # 行0随窗口大小改变而自适应
root.bind("<Configure>", app.resize) # 监听窗口大小变化
root.mainloop()
```
在上面的例子中,我们使用 `grid` 方法将标签置于第一行第一列,然后使用 `resize` 方法监听窗口大小变化,然后根据窗口的宽度和高度计算出新的字体大小,并更新标签的字体。
3. `pack` 方法:可以将控件放置在窗口的顶部、底部或中间等位置,通过改变控件的尺寸和位置,让控件随窗口大小改变而自适应缩放。
```python
import tkinter as tk
class App:
def __init__(self, master):
self.master = master
self.label = tk.Label(master, text="Hello World", font=("Arial", 12))
self.label.pack(fill=tk.BOTH, expand=True)
# 将标签填充整个窗口并自适应大小
def resize(self, event):
w, h = event.width, event.height
size = int((w + h) / 30)
self.label.config(font=("Arial", size))
root = tk.Tk()
app = App(root)
root.bind("<Configure>", app.resize) # 监听窗口大小变化
root.mainloop()
```
在上面的例子中,我们使用 `pack` 方法将标签填充整个窗口并自适应大小,然后使用 `resize` 方法监听窗口大小变化,然后根据窗口的宽度和高度计算出新的字体大小,并更新标签的字体。
使用这些方法可以让控件根据屏幕大小自适应缩放,从而达到更好的用户体验。
阅读全文
相关推荐
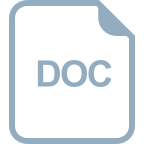
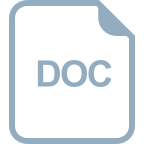
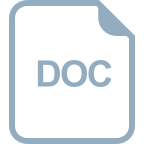
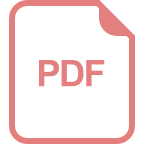
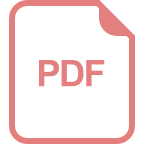
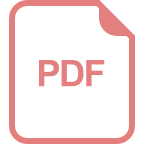
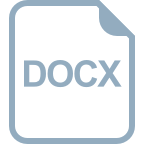
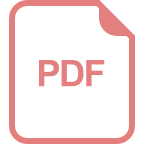
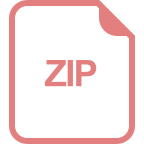
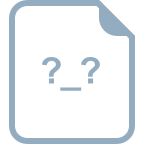
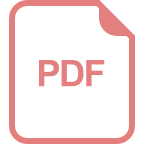
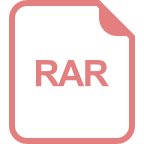
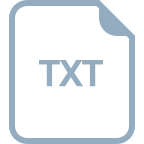
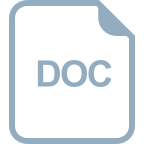
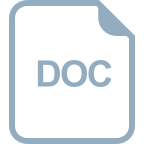
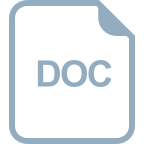
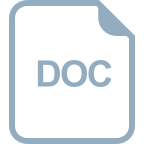