用整型数组表示10进制大整数(超过2^32的整数),数组的每个元素存储大整数的一位数字,实现大整数的加减法。使用c++
时间: 2024-03-18 18:38:37 浏览: 45
以下是一个简单的实现,仅供参考:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class BigInt {
private:
vector<int> digits; // 用 vector 存储每一位数字
public:
BigInt() {} // 默认构造函数
BigInt(int num) { // 构造函数,将一个整数转换为大整数
while (num) {
digits.push_back(num % 10);
num /= 10;
}
}
BigInt(const string& str) { // 构造函数,将一个字符串转换为大整数
for (int i = str.length() - 1; i >= 0; i--) {
digits.push_back(str[i] - '0');
}
}
BigInt operator+(const BigInt& other) const { // 大整数加法
BigInt result;
int carry = 0;
int i = 0, j = 0;
while (i < digits.size() || j < other.digits.size() || carry) {
int sum = carry;
if (i < digits.size()) {
sum += digits[i++];
}
if (j < other.digits.size()) {
sum += other.digits[j++];
}
result.digits.push_back(sum % 10);
carry = sum / 10;
}
return result;
}
BigInt operator-(const BigInt& other) const { // 大整数减法
BigInt result;
int borrow = 0;
int i = 0, j = 0;
while (i < digits.size() || j < other.digits.size()) {
int diff = borrow;
if (i < digits.size()) {
diff += digits[i++];
}
if (j < other.digits.size()) {
diff -= other.digits[j++];
}
if (diff < 0) {
diff += 10;
borrow = -1;
} else {
borrow = 0;
}
result.digits.push_back(diff);
}
while (result.digits.size() > 1 && result.digits.back() == 0) {
result.digits.pop_back();
}
return result;
}
friend ostream& operator<<(ostream& os, const BigInt& num) { // 重载输出运算符
for (int i = num.digits.size() - 1; i >= 0; i--) {
os << num.digits[i];
}
return os;
}
};
int main() {
BigInt a("123456789012345678901234567890");
BigInt b("987654321098765432109876543210");
BigInt c = a + b;
cout << c << endl;
BigInt d = b - a;
cout << d << endl;
return 0;
}
```
这个实现中,我们使用了 `vector<int>` 来存储大整数的每一位数字。在构造函数中,我们将一个整数或字符串转换为大整数。在加法和减法操作中,我们使用类似手工计算的方法,从低位到高位依次计算每一位的和或差,并考虑进位和借位。在输出运算符中,我们将大整数倒序输出。
阅读全文
相关推荐





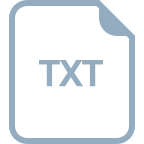
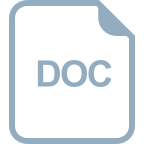
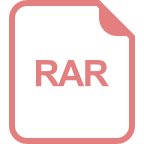
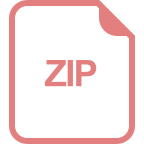
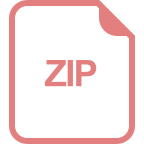
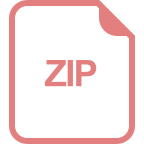
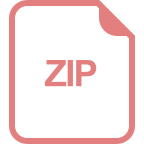