将M行N 列的二维数组中的数据,按行的顺序依次存放到一维数组中,一维数组中数据的个数存放在形参n所指的存储单元中
时间: 2023-05-21 14:04:41 浏览: 146
可以使用双重循环,将二维数组中的每一个元素逐一存放到一维数组中,直到一维数组存储满了所有的数据。具体实现代码如下:
void twoDArrayToOneDArray(int **twoDArray, int M, int N, int *oneDArray, int *n)
{
int count = 0;
for (int i = 0; i < M; i++) {
for (int j = 0; j < N; j++) {
if (count < *n) {
oneDArray[count++] = twoDArray[i][j];
} else {
return;
}
}
}
}
其中,twoDArray为二维数组的指针,M和N分别表示二维数组的行数和列数,oneDArray为一维数组的指针,n为一维数组的最大容量。函数执行完之后,n所指向的存储单元中将保存一维数组中实际存储的数据个数。
相关问题
请编写函数fun(),其功能是将m行n列的二维数组中的数据按行的顺序依次存放到一维数
```python
def fun(arr, m, n):
result = []
for i in range(m):
for j in range(n):
result.append(arr[i][j])
return result
```
这段代码定义了一个名为fun的函数,接受一个二维数组arr以及其行数m和列数n作为参数。然后使用两层循环遍历二维数组中的元素,按行的顺序依次存放到一维数组result中。最后返回这个一维数组作为结果。
你可以将你的二维数组和其行列数传递给这个函数,它将返回按行顺序排列的一维数组。
C语言,将N×N的二维数组中的字符数据按列的顺序依次放到一个字符串(一维数组)中
可以按照如下步骤进行操作:
1. 定义一个一维字符数组,其长度为N*N,用于存放二维数组中的字符数据。
2. 使用双重循环遍历二维数组中的每一个元素,将其按列的顺序依次放入一维数组中。
3. 循环结束后,一维数组中的数据即为按列顺序存储的字符数据。
下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 3 // 定义二维数组的大小
int main()
{
char a[N][N] = {{'a', 'b', 'c'},
{'d', 'e', 'f'},
{'g', 'h', 'i'}}; // 定义二维字符数组
char* str = (char*)malloc(sizeof(char) * N * N); // 定义一维字符数组
int k = 0; // 记录一维数组中下一个可以存储的位置
// 将二维数组中的数据按列顺序存储到一维数组中
for (int j = 0; j < N; j++) {
for (int i = 0; i < N; i++) {
str[k++] = a[i][j];
}
}
// 输出存储结果
printf("Result: %s\n", str);
// 释放内存
free(str);
return 0;
}
```
输出结果为:
```
Result: adgbehcfi
```
相关推荐
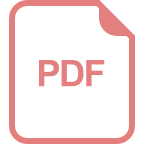
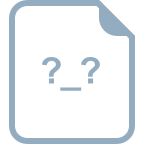
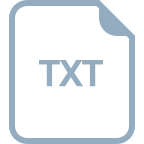












