下面这个方法中每一个函数的作用:def show_cv_img(self, img): shrink = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) QtImg = QtGui.QImage(shrink.data, shrink.shape[1], shrink.shape[0], shrink.shape[1] * 3, QtGui.QImage.Format_RGB888) jpg_out = QtGui.QPixmap(QtImg).scaled( self.label.width(), self.label.height()) self.label.setPixmap(jpg_out)
时间: 2023-06-07 18:11:40 浏览: 216
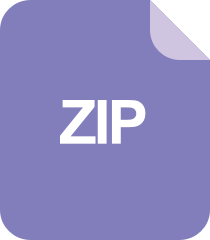
shrink_zoom_bilinear.zip_bilinear shrink_zoom
好的,我会回答你的问题。这个方法主要是用来将 opencv 格式的图片转化为 Qt 格式的图片,并将转化后的图片显示在 GUI 上。下面是每一个函数的作用:
- cv2.cvtColor(img, cv2.COLOR_BGR2RGB):将 BGR 格式的图片转化为 RGB 格式。
- QtGui.QImage():将转化后的图片数据包装为 QImage 对象。
- QtGui.QPixmap():将 QImage 对象转化为 QPixmap 对象,并进行缩放操作。
- self.label.setPixmap():将缩放后的 QPixmap 赋值给 QLabel 对象,用于在 GUI 上显示图片。
阅读全文
相关推荐
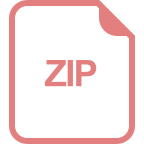
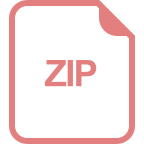















